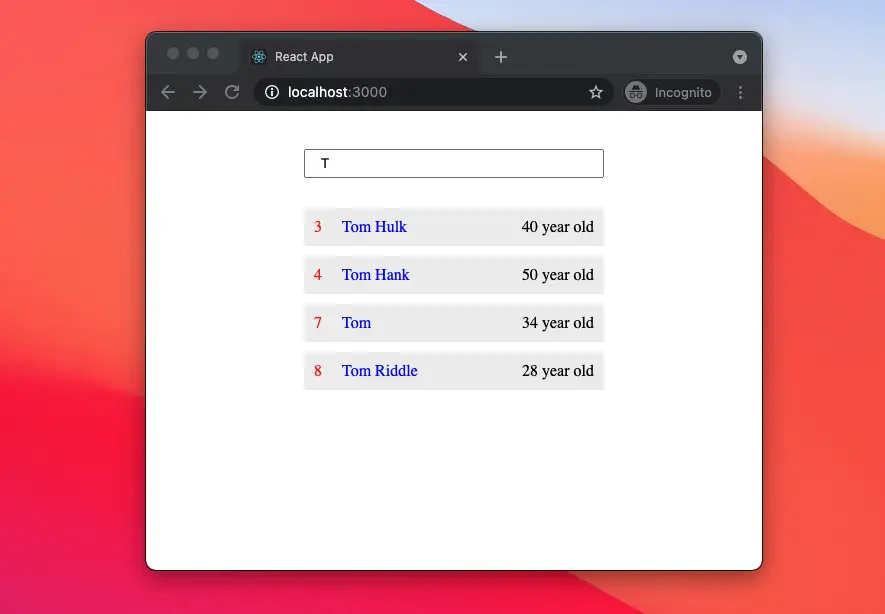
This article walks you through a complete example of making a filter (searchable) list in React. We’ll use the most recent stable version of React (18.3) as well as use functional components and hooks.
Table of Contents
The Example
Let’s say we have a list of users. Our job is to filter (search) some users by their names.
We’ll use the filter() and startsWith() methods (just two Javascript methods) to find out users whose names match the text entered in the search box. We also use the toLowerCase() method to make it case-insensitive.
When the search box is empty, all users will be displayed.
Preview
Javascript code
To make sure we start at the same place, you should create a brand new React project:
npx create-react-app kindacode-example
Here’s the full source code in src/App.js:
import React, { useState } from 'react';
import './App.css';
// This holds a list of some fiction people
// Some have the same name but different age and id
const USERS = [
{ id: 1, name: 'Andy', age: 32 },
{ id: 2, name: 'Bob', age: 30 },
{ id: 3, name: 'Tom Hulk', age: 40 },
{ id: 4, name: 'Tom Hank', age: 50 },
{ id: 5, name: 'Audra', age: 30 },
{ id: 6, name: 'Anna', age: 68 },
{ id: 7, name: 'Tom', age: 34 },
{ id: 8, name: 'Tom Riddle', age: 28 },
{ id: 9, name: 'Bolo', age: 23 },
];
function App() {
// the value of the search field
const [name, setName] = useState('');
// the search result
const [foundUsers, setFoundUsers] = useState(USERS);
const filter = (e) => {
const keyword = e.target.value;
if (keyword !== '') {
const results = USERS.filter((user) => {
return user.name.toLowerCase().startsWith(keyword.toLowerCase());
// Use the toLowerCase() method to make it case-insensitive
});
setFoundUsers(results);
} else {
setFoundUsers(USERS);
// If the text field is empty, show all users
}
setName(keyword);
};
return (
<div className="container">
<input
type="search"
value={name}
onChange={filter}
className="input"
placeholder="Filter"
/>
<div className="user-list">
{foundUsers && foundUsers.length > 0 ? (
foundUsers.map((user) => (
<li key={user.id} className="user">
<span className="user-id">{user.id}</span>
<span className="user-name">{user.name}</span>
<span className="user-age">{user.age} year old</span>
</li>
))
) : (
<h1>No results found!</h1>
)}
</div>
</div>
);
}
export default App;
CSS code
Below’s the full source code in src/App.css:
.container {
padding-top: 30px;
width: 300px;
margin: auto;
}
.input {
padding: 5px 15px;
width: 300px;
}
.user-list {
margin-top: 30px;
}
.user {
list-style: none;
margin: 10px 0;
padding: 10px;
background: #eee;
display: flex;
justify-content: space-between;
}
.user-id {
color: red;
margin-right: 20px;
}
.user-name {
color: blue;
}
.user-age {
margin-left: auto;
}
Conclusion
Congratulation! At this point, you should get a better understanding and feel more comfortable when working with a filter/search list in React. Continue learning and pushing forward by taking a look at the following articles:
- React useReducer hook – Tutorial and Examples
- How to fetch data from APIs with Axios and Hooks in React
- Most popular React Component UI Libraries
- React + TypeScript: Making a Custom Context Menu
- React + TypeScript: Handling onFocus and onBlur events
- React: How to Upload Multiple Files with Axios
- React: Create an Animated Side Navigation from Scratch
You can also check our React topic page and React Native topic page for the latest tutorials and examples.