To create perfectly round images in React Native, just give the borderRadius property a very high value.
Example
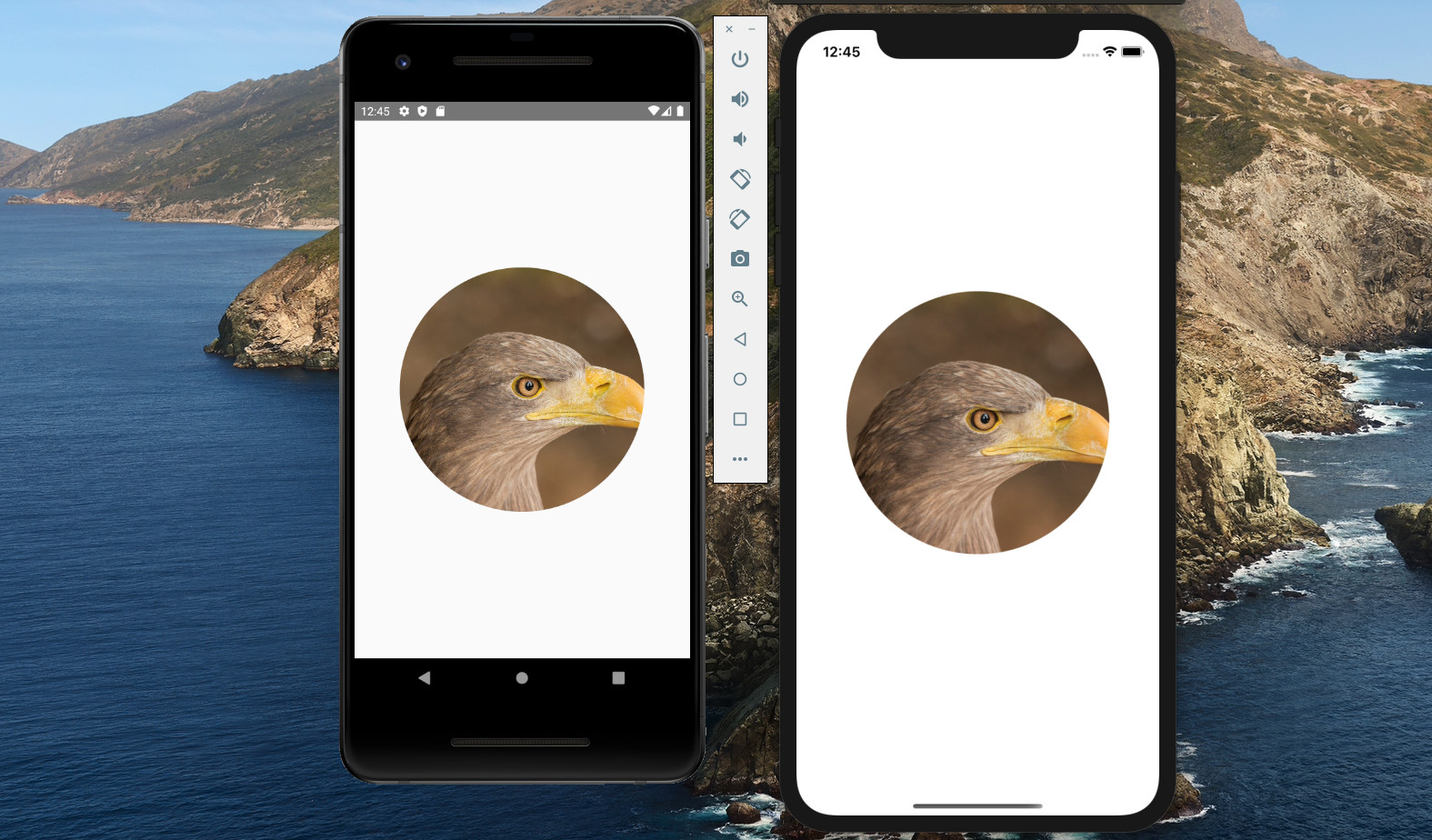
The code:
// App.js
import React from "react";
import { View, StyleSheet, Image } from "react-native";
const IMG_URI =
"https://cdn.pixabay.com/photo/2020/05/26/15/42/eagle-5223559_960_720.jpg";
function App() {
return (
<View style={styles.screen}>
<Image style={styles.image} source={{ uri: IMG_URI }} />
</View>
);
}
// Kindacode.com
// Just some styles
const styles = StyleSheet.create({
screen: {
flex: 1,
justifyContent: "center",
alignItems: "center",
},
// styling the image
image: {
width: 300,
height: 300,
borderRadius: 1000,
},
});
export default App;
That’s it. Further reading:
- How to Get the Window Width & Height in React Native
- How to Implement a Picker in React Native
- React Native FlatList: Tutorial and Examples
- How to render HTML content in React Native
- How to implement tables in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.