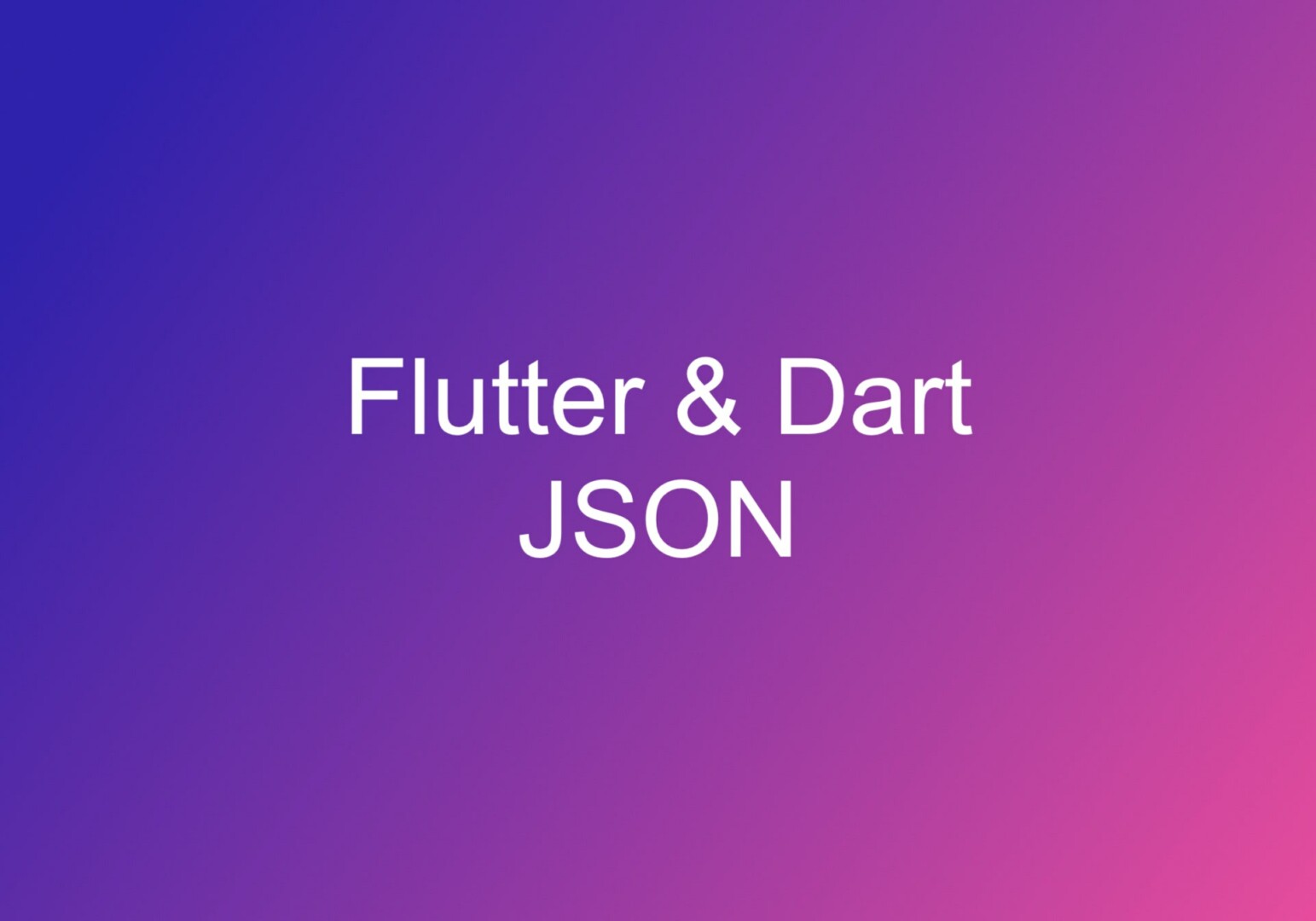
Table of Contents
A Quick Note
This article shows you how to encode/decode JSON in Flutter. The steps you need to follow are:
1. Import the dart:convert library:
import 'dart:convert';
2. Use:
- json.encode() or jsonEncode() for encoding.
- json.decode() or jsonDecode() for decoding.
The concepts are just brief. Now, it’s time for us to take a look at some code examples to understand and master them.
Examples
Example 1: JSON Encoding
import 'dart:convert';
void main() {
final products = [
{'id': 1, 'name': 'Product #1'},
{'id': 2, 'name': 'Product #2'}
];
print(json.encode(products));
}
Output:
[{"id":1,"name":"Product #1"},{"id":2,"name":"Product #2"}]
Example 2: JSON decoding
import 'dart:convert';
import 'package:flutter/foundation.dart';
void main() {
const String responseData =
'[{"id":1,"name":"Product #1"},{"id":2,"name":"Product #2"}]';
final products = json.decode(responseData);
if (kDebugMode) {
// Print the type of "products"
print(products.runtimeType);
// Print the name of the second product in the list
print(products[1]['name']);
}
}
Output:
List<dynamic>
Product #2
Hope this helps. Further reading:
- How to read data from local JSON files in Flutter
- Ways to Fetch Data from APIs in Flutter
- Best Libraries for Making HTTP Requests in Flutter
- Flutter: Firebase Remote Config example
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.