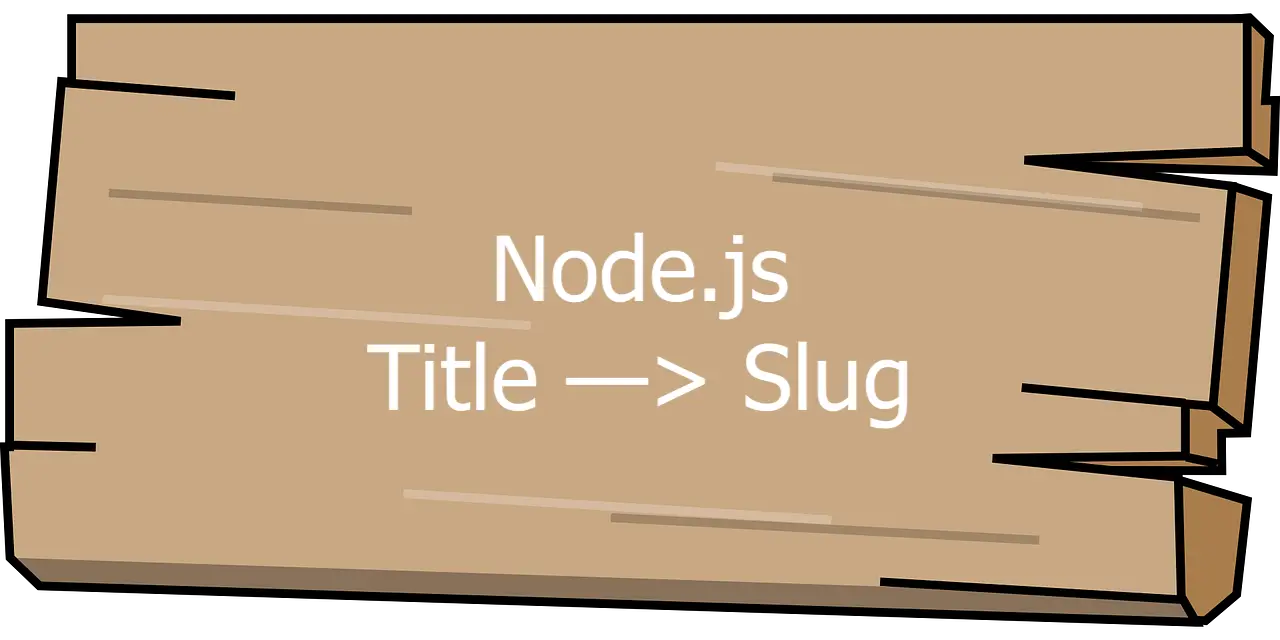
This article shows you a few approaches to generating a slug from a title in Node.js. The first one is to use self-written code and the other is to use a third-party package.
Introduction
A slug is the part of a URL that describes something about a webpage’s content. For example, we have a URL like this:
https://www.kindacode.com/article/how-to-generate-slugs-from-titles-in-node-js
Then the slug is:
how-to-generate-slugs-from-titles-in-node-js
A slug often contains only some “friendly” characters in lower case: a-z, 0-9, and the dash symbol (some sites prefer the underscore over the dash). This is not a strict rule but is widely used by most English and Latin-based-language news websites: CNN, The New York Times, The Guardian…
Using self-written code
A slug of a page is ordinarily generated from this page’s title. And, the example below demonstrates how to get this matter done by using Node.js:
// kindacode.com
const titleToSlug = title => {
let slug;
// convert to lower case
slug = title.toLowerCase();
// remove special characters
slug = slug.replace(/\`|\~|\!|\@|\#|\||\$|\%|\^|\&|\*|\(|\)|\+|\=|\,|\.|\/|\?|\>|\<|\'|\"|\:|\;|_/gi, '');
// The /gi modifier is used to do a case insensitive search of all occurrences of a regular expression in a string
// replace spaces with dash symbols
slug = slug.replace(/ /gi, "-");
// remove consecutive dash symbols
slug = slug.replace(/\-\-\-\-\-/gi, '-');
slug = slug.replace(/\-\-\-\-/gi, '-');
slug = slug.replace(/\-\-\-/gi, '-');
slug = slug.replace(/\-\-/gi, '-');
// remove the unwanted dash symbols at the beginning and the end of the slug
slug = '@' + slug + '@';
slug = slug.replace(/\@\-|\-\@|\@/gi, '');
return slug;
};
// Testing
const title = "This is a special title, isn't it?";
const slug = titleToSlug(title);
console.log(slug);
Output:
this-is-a-special-title-isnt-it
The code snippet above works well for English titles. If you’re using other languages like Spanish, French, or Portuguese… then you may need some extra improvements.
Note: Most Chinese, Korean, and Japanese websites don’t use slugs in their URLs (generally, they only use IDs in URLs).
Using a third-party package
There are several open-source packages that can help us get the job done quickly such as slugify, slug, github-slugger, etc. In the following example, we will use slugify, a lightweight, and widely used library.
Add slugify to your Node.js project by running:
npm install slugify --save
Example:
// import it
// if your project goes with CommonJS, use "require" instead
import slugify from "slugify";
// Simple use
const title1 =
'He thrusts his fists against the posts and still insists he sees the ghosts';
const slug1 = slugify(title1);
console.log(slug1);
// Config your options
const options = {
replacement: '-', // replace spaces with replacement character, defaults to `-`
remove: undefined, // remove characters that match regex, defaults to `undefined`
lower: true, // convert to lower case, defaults to `false`
strict: true, // strip special characters except replacement, defaults to `false`
locale: 'en', // language code of the locale to use
};
const title2 = 'The Man in the Wind @@!** and the Dog';
const slug2 = slugify(title2, options);
console.log(slug2);
Output:
He-thrusts-his-fists-against-the-posts-and-still-insists-he-sees-the-ghosts
the-man-in-the-wind-and-the-dog
Conclusion
You’ve learned more than one technique to create a slug from a title in Node.js. This knowledge is really helpful when developing backends for blogs, news, e-commerce websites, or other kinds of applications. If you’d like to explore more interesting stuff, take a look at the following articles:
- 6 best Node.js frameworks to build backend APIs
- Top 4 best Node.js Open Source Headless CMS
- Using Axios to download images and videos in Node.js
- Node.js: Get domain, hostname, and protocol from a URL
- Node.js: Listing Files in a Folder
- Node.js: The Maximum Size Allowed for Strings/Buffers
You can also check out our Node.js category page for the latest tutorials and examples.