This article shows you how to handle error network images in Flutter.
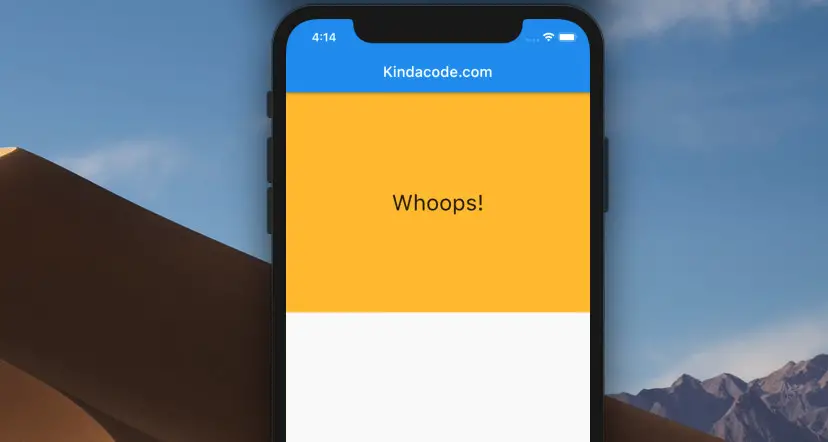
If you use Image.network() to display an online image and that image is broken (404 not found, 403 forbidden, etc.), you can use the errorBuilder property to render something instead, such as a default image, text…
Example
This code snippet produces the app that is displayed in the screenshot above:
SizedBox(
width: double.infinity,
height: 300,
child: Image.network(
'https://www.kindacode.com/no-image.jpg', // this image doesn't exist
fit: BoxFit.cover,
errorBuilder: (context, error, stackTrace) {
return Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'Whoops!',
style: TextStyle(fontSize: 30),
),
);
},
),
),
Complete source code (including boilerplate):
// kindacode.com
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
// hide debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
home: KindaCodeDemo(),
);
}
}
class KindaCodeDemo extends StatefulWidget {
const KindaCodeDemo({super.key});
@override
State<KindaCodeDemo> createState() => _KindaCodeDemoState();
}
class _KindaCodeDemoState extends State<KindaCodeDemo> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: SizedBox(
width: double.infinity,
height: 300,
child: Image.network(
'https://www.kindacode.com/no-image.jpg', // this image doesn't exist
fit: BoxFit.cover,
errorBuilder: (context, error, stackTrace) {
return Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'Whoops!',
style: TextStyle(fontSize: 30),
),
);
},
),
),
);
}
}
I hope this helps.
Further reading:
- Flutter: Create a Password Strength Checker from Scratch
- Flutter: Caching Network Images for Big Performance gains
- Flutter: Reading Bytes from a Network Image
- Flutter: Set an image Background for the entire screen
- Flutter: Display Text over Image without using Stack widget
- Flutter: Drawing an N-Pointed Star with CustomClipper
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.