This article will show you how to use Font Awesome icons in a React app.
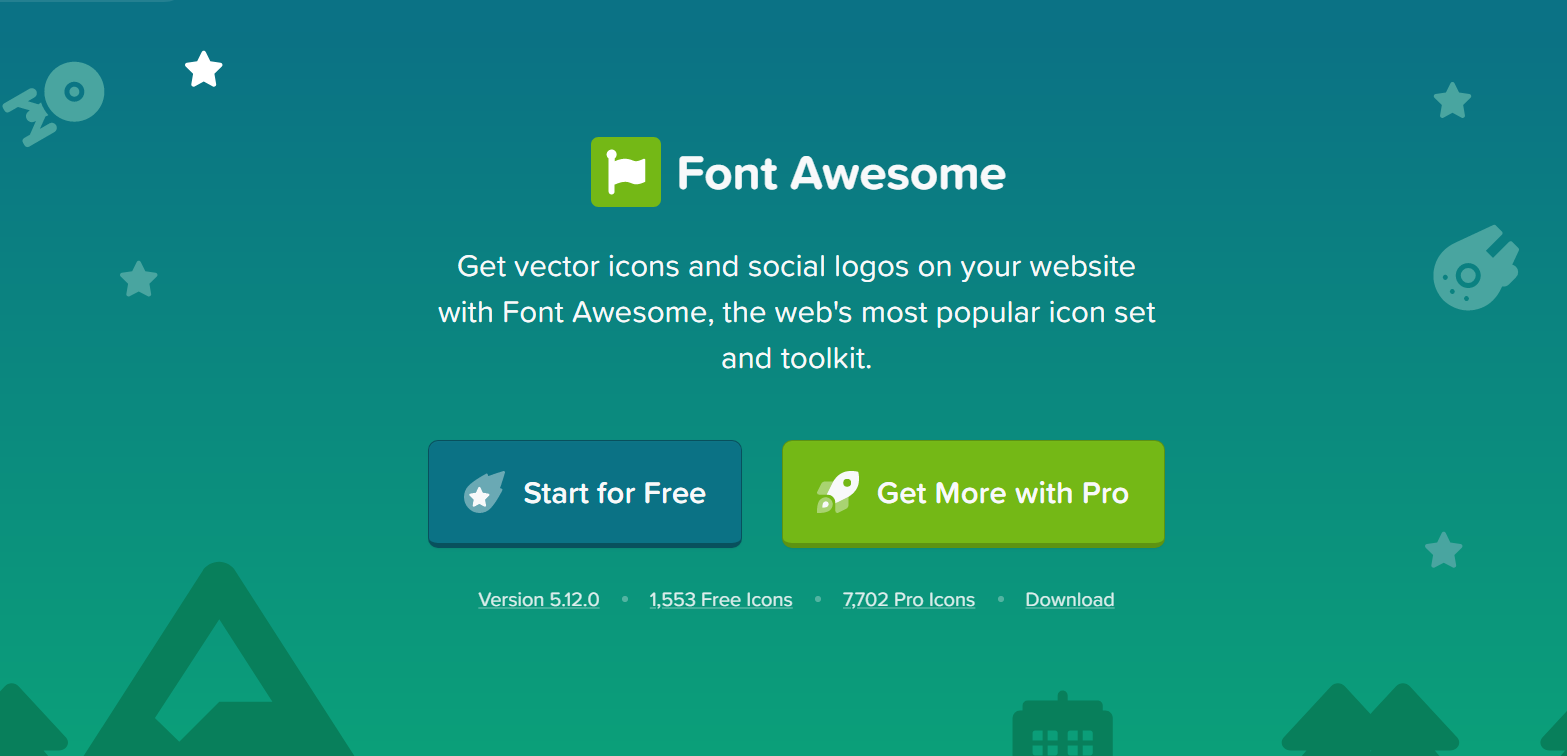
Firstly, let’s install the necessary packages:
npm i @fortawesome/react-fontawesome
npm i @fortawesome/free-solid-svg-icons
npm i @fortawesome/fontawesome-svg-core
Note that you can also install all of the packages above by running this “one-shot” command only:
npm i @fortawesome/react-fontawesome @fortawesome/free-solid-svg-icons @fortawesome/fontawesome-svg-core
Next, import the icons you need and use them (and style them) in your React component:
import React from "react";
import { FontAwesomeIcon } from "@fortawesome/react-fontawesome";
import {
faClock, // the clock icon
faUserCircle, // the user circle icon
faCoffee, // a cup of coffee
} from "@fortawesome/free-solid-svg-icons";
const MyComponent = () => {
return (
<div style={{ padding: 50 }}>
<p>
<FontAwesomeIcon
icon={faClock}
style={{ fontSize: 100, color: "blue", marginRight: 30 }}
/>
<FontAwesomeIcon
icon={faUserCircle}
style={{ fontSize: 100, color: "orange" }}
/>
</p>
<p>
<FontAwesomeIcon
icon={faCoffee}
style={{ fontSize: 200, color: "brown" }}
/>
</p>
</div>
);
};
export default MyComponent;
Output:
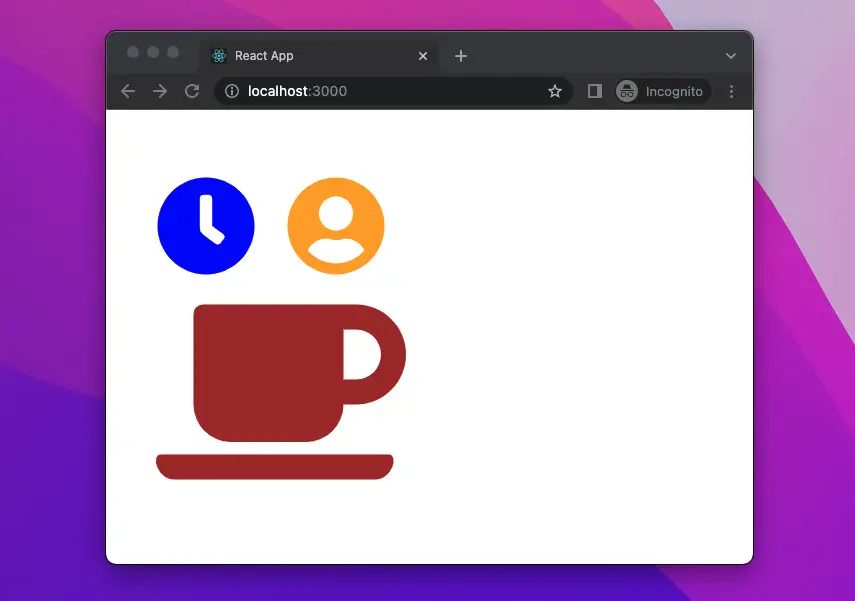
You can find all available icons in the following file:
<your project>/node_modules/@fortawesome/free-solid-svg-icons/index.d.ts
That’s it. Further reading:
- React Router Dom: Implement a Not Found (404) Route
- How to Use Tailwind CSS in React
- How to Use Bootstrap 5 and Bootstrap Icons in React
- React Router Dom: Scroll To Top on Route Change
- React + TypeScript: onMouseOver & onMouseOut events
- React + TypeScript: Handling onFocus and onBlur events
You can also check our React topic page and React Native topic page for the latest tutorials and examples.