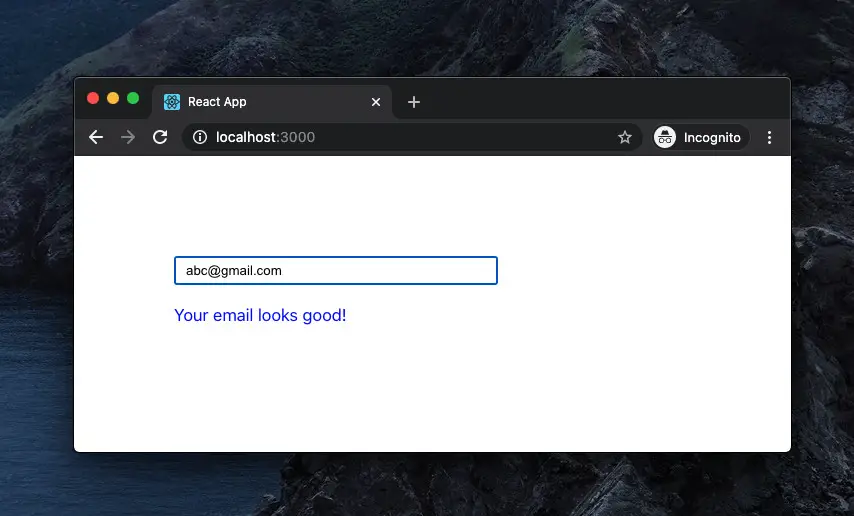
This article shows you how to validate email addresses while the user is typing in React (live validation).
Table of Contents
Summary
Here’re the points:
- We’ll use Javascript regular expression so that no third-party library is required.
- We’ll use the functional component and the useState hook.
- Frontend validation is never secure enough, so you (or your team) need to implement server-side validation later.
The Steps
1. Create a new React app by running:
npx create-react-app live_email_validation
2. Remove all the default code in App.js and add this:
// KindaCode.com
// App.js
import React, { useState } from 'react';
import './App.css';
function App() {
const [isValid, setIsValid] = useState(false);
const [message, setMessage] = useState('');
// The regular exprssion to validate the email pattern
// It may not be 100% perfect but can catch most email pattern errors and assures that the form is mostly right
const emailRegex = /\S+@\S+\.\S+/;
const validateEmail = (event) => {
const email = event.target.value;
if (emailRegex.test(email)) {
setIsValid(true);
setMessage('Your email looks good!');
} else {
setIsValid(false);
setMessage('Please enter a valid email!');
}
};
return (
<div className="container">
<input
type="email"
placeholder="Enter your email"
className="email-input"
onChange={validateEmail}
/>
{/*If the entered email is valid, the message will be blue, otherwise it will be red. */}
<div className={`message ${isValid ? 'success' : 'error'}`}>
{message}
</div>
</div>
);
}
export default App;
3. Replace the default code in App.css with this:
.container {
padding: 100px;
}
.email-input {
width: 300px;
padding: 5px 10px;
}
.message {
margin-top: 20px;
font-size: 17px;
}
.success {
color: blue;
}
.error {
color: red;
}
4. Try it by running:
npm start
And here’s what you’ll see when going to http://localhost:3000 in the browser:
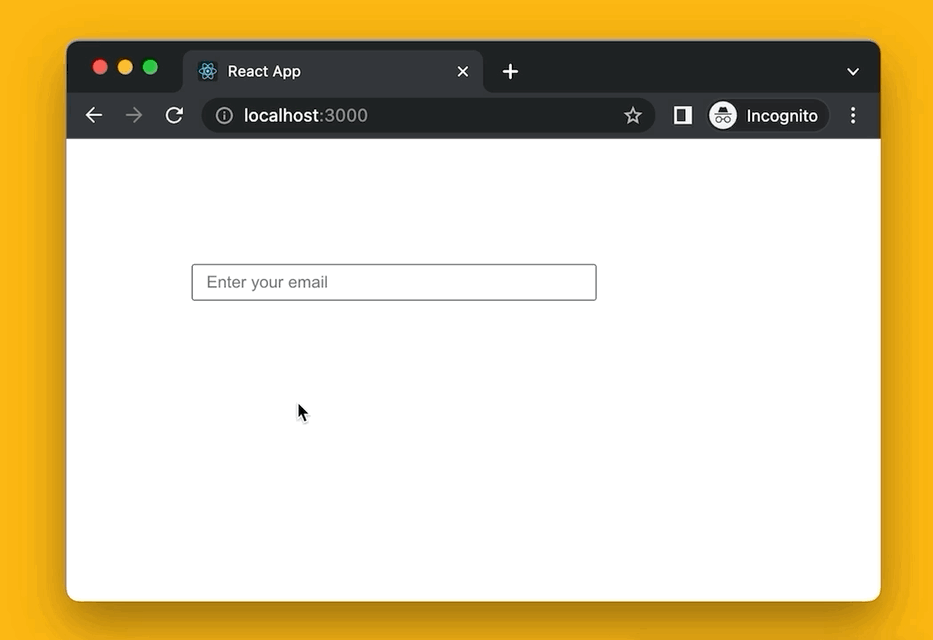
Conclusion
We’ve walked through a complete example of validating user email in real time. If you’d like to explore more new and exciting things in the modern React world, take a look at the following articles:
- React Router Dom: Implement a Not Found (404) Route
- React + TypeScript: Making a Custom Context Menu
- React: Show Image Preview before Uploading
- React + TypeScript: Working with Radio Button Groups
- React: Using inline styles with the calc() function
- React: Create an Animated Side Navigation from Scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.