A simple example of using a loading indicator in Flutter.
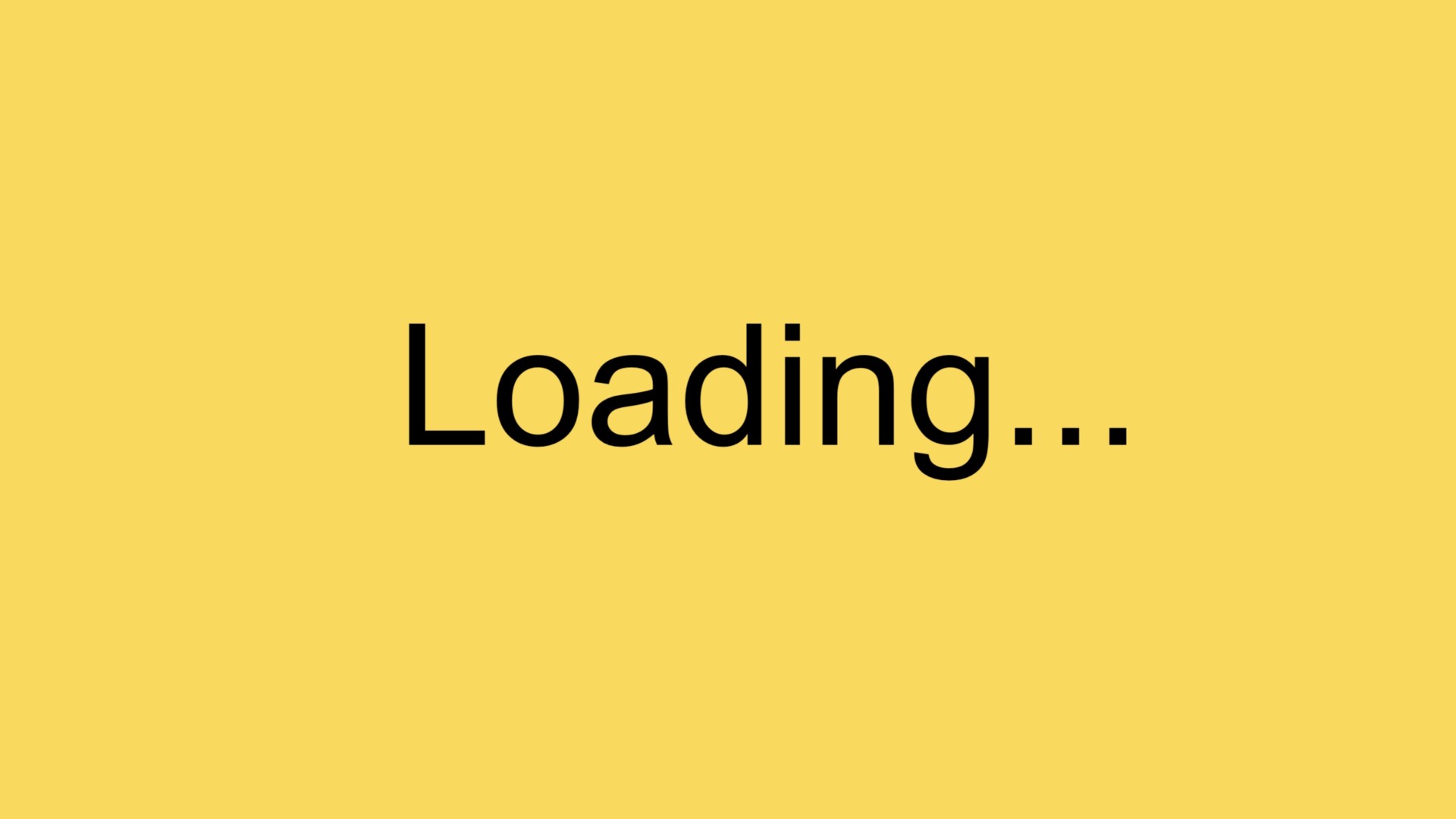
We’ll make a tiny Flutter app that contains a button. When the user clicks that button, a loading indicator will show up for 5 seconds and then disappear.
The code:
import 'dart:async';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
bool _isLoading = false;
// This function will be called when the button gets pressed
_startLoading() {
setState(() {
_isLoading = true;
});
Timer(const Duration(seconds: 5), () {
setState(() {
_isLoading = false;
});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: const Text(
'Kindacode.com',
),
),
body: Center(
child: _isLoading
? const CircularProgressIndicator()
: ElevatedButton(
onPressed: _startLoading, child: const Text('Start Loading')),
));
}
}
Output:
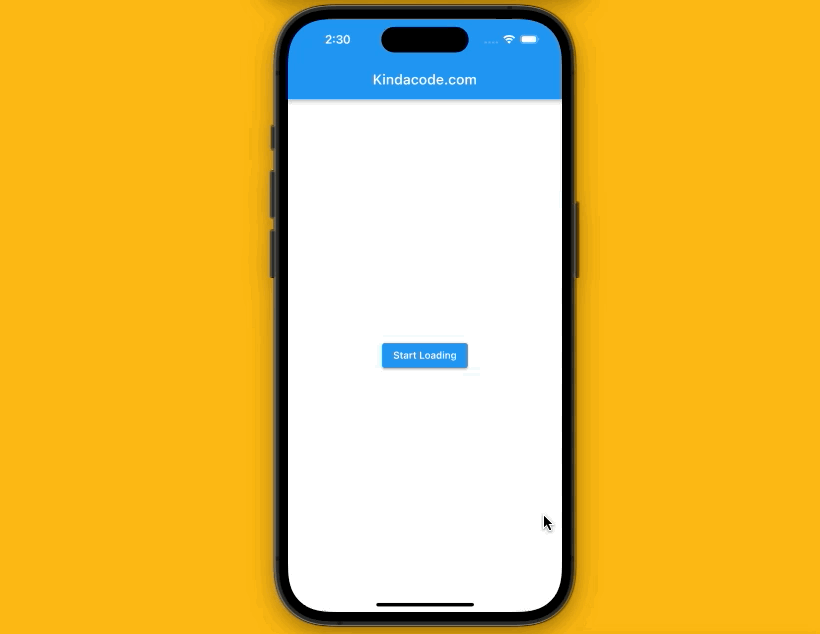
Hope this helps. Futher reading:
- React Native: Make a Button with a Loading Indicator inside
- 2 Ways to Fetch Data from APIs in Flutter
- Viewing PDF files in Flutter
- Flutter: Reading Bytes from a Network Image
- Flutter image loading builder example
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.