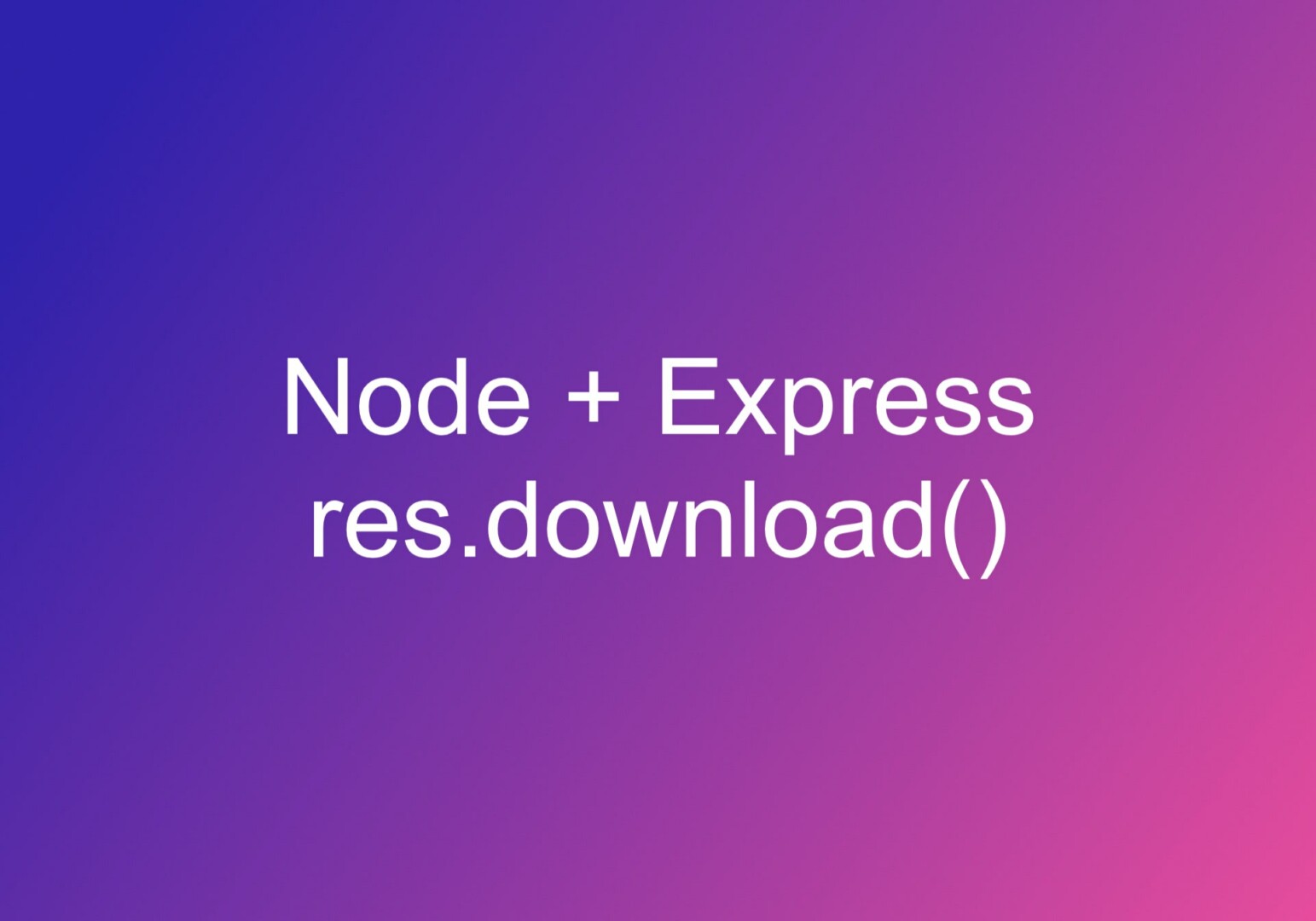
The example below shows you how to automatically download an image served from Express server when clicking a link.
1. Navigate to the folder you want your project to lie in then run:
npm init
2. Install express:
npm i express
3. Create a new folder named files. Copy into this folder an image named test.jpg. You can use an image from your computer or download the sample from here:
https://www.kindacode.com/wp-content/uploads/2020/04/test.jpeg
Our project structure:
.
├── files
│ └── test.jpg
├── index.js
├── node_modules
├── package-lock.json
└── package.json
4. Add the following code to index.js:
const path = require("path");
const express = require("express");
const app = express();
const port = 3000;
app.use(express.static(path.join(__dirname, "files")));
const fileName = 'test.jpg';
const fileUrl = `http://localhost:${port}/${fileName}`;
const filePath = path.join(__dirname, 'files', fileName);
app.get("/", (req, res, next) => {
res.send(`
<img src=${fileUrl} />
<br>
<br>
<a href="/download">Download</a>
`);
res.end();
});
app.get("/download", (req, res, next) => {
res.download(filePath);
})
app.listen(port);
5. Get our project up and running by executing the following command:
node app.js
Open http://localhost:3000 with your browser and hit “Download” to see what happens:
That’s it. Further reading:
- Node + TypeScript: Export Default Something based on Conditions
- Express + TypeScript: Extending Request and Response objects
- Using Axios to download images and videos in Node.js
- 2 Ways to Set Default Time Zone in Node.js
- Node.js: How to Compress a File using Gzip format
You can also check out our Node.js category page for the latest tutorials and examples.