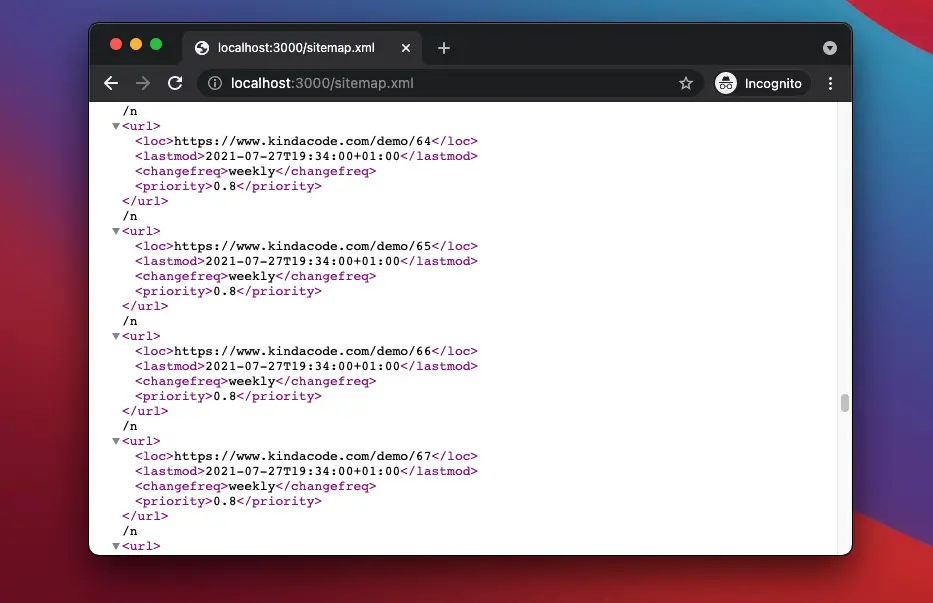
This article walks you through a few examples of responding in XML when using Express and Node.js. Besides express, we don’t need any other npm libraries.
Example 1: Basic
This example creates a list of fiction products and returns it in XML format instead of JSON.
The complete code
// kindacode.com
// index.js
import express from 'express'
const app = express();
app.get("/", (req, res, next) => {
let data = `<?xml version="1.0" encoding="UTF-8"?>`;
data += `<products>`;
for (let i = 0; i < 100; i++) {
data += `<item>
<name>Product ${i}</name>
<price>${i}</price>
</item>`;
}
data += `</products>`;
res.header("Content-Type", "application/xml");
res.status(200).send(data);
});
app.listen(3000, () => {
console.log("Server is running on port 3000");
});
Run it:
node index.js
Or:
nodemon index.js
Check The Result
Open your web browser and navigate to http://localhost:3000 then you’ll see something like this:
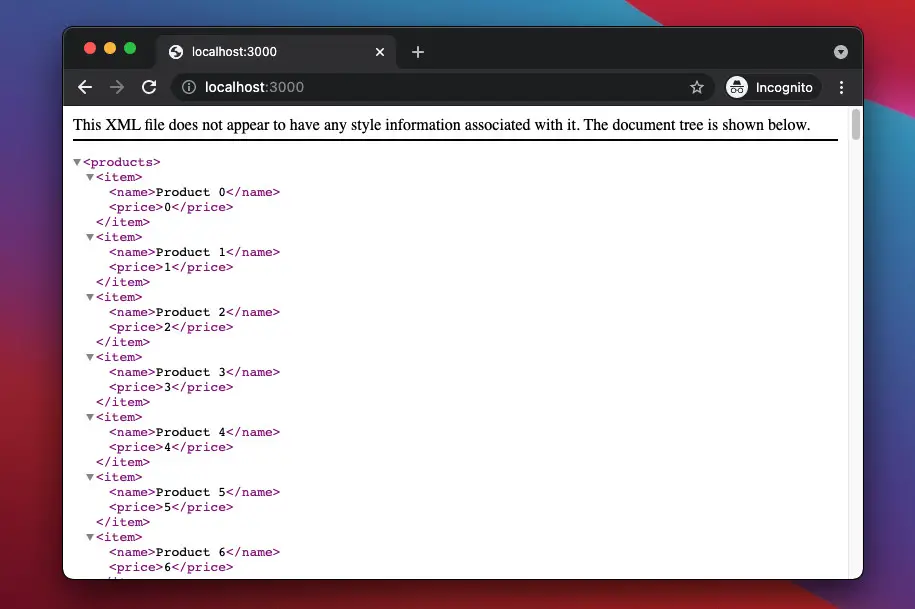
Example 2: Making an XML Sitemap
This example is a real-world use case: making an XML sitemap.
The code
import express from 'express';
const app = express();
app.get("/sitemap.xml", (req, res, next) => {
let data = `<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
`;
const urls = [];
urls.push(`<url>
<loc>https://www.kindacode.com</loc>
<changefreq>dailly</changefreq>
<priority>1.0</priority>
</url>`);
// Fetch your urls from database or somewhere else
// The add to the urls array
for (let i = 0; i < 100; i++) {
urls.push(`<url>
<loc>https://www.kindacode.com/demo/${i}</loc>
<lastmod>2021-07-27T19:34:00+01:00</lastmod>
<changefreq>weekly</changefreq>
<priority>0.8</priority>
</url>`);
}
data += urls.join("/n");
data += `</urlset>`;
res.header("Content-Type", "application/xml");
res.status(200).send(data);
});
app.listen(3000, () => {
console.log("KindaCode server is running on port 3000");
});
Output
Open your web browser and navigate to http://localhost/sitemap.xml. You should see something like this:
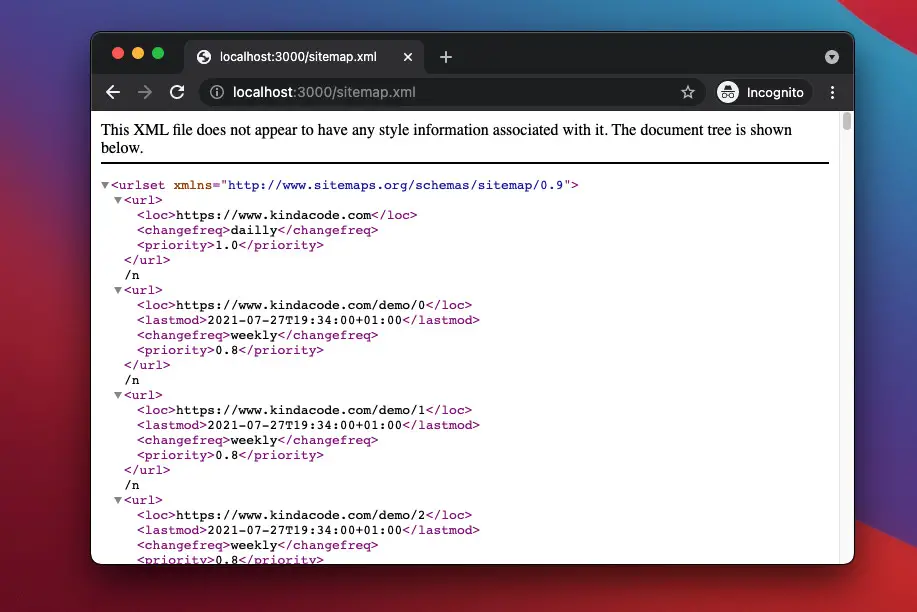
Conclusion
We’ve examined a couple of examples of responding XML data using Node.js and Express.js. If you’d like to explore more modern and fascinating stuff about backend development with Javascript, take a look at the following articles:
- How to Delete a File or Directory in Node.js
- 4 Ways to Convert Object into Query String in Node.js
- Top 5 best Node.js Open Source Headless CMS
- 7 best Node.js frameworks to build backend APIs
- Using Axios to download images and videos in Node.js
- 7 Best Open-Source HTTP Request Libraries for Node.js
You can also check out our Node.js category page or PHP category page for the latest tutorials and examples.