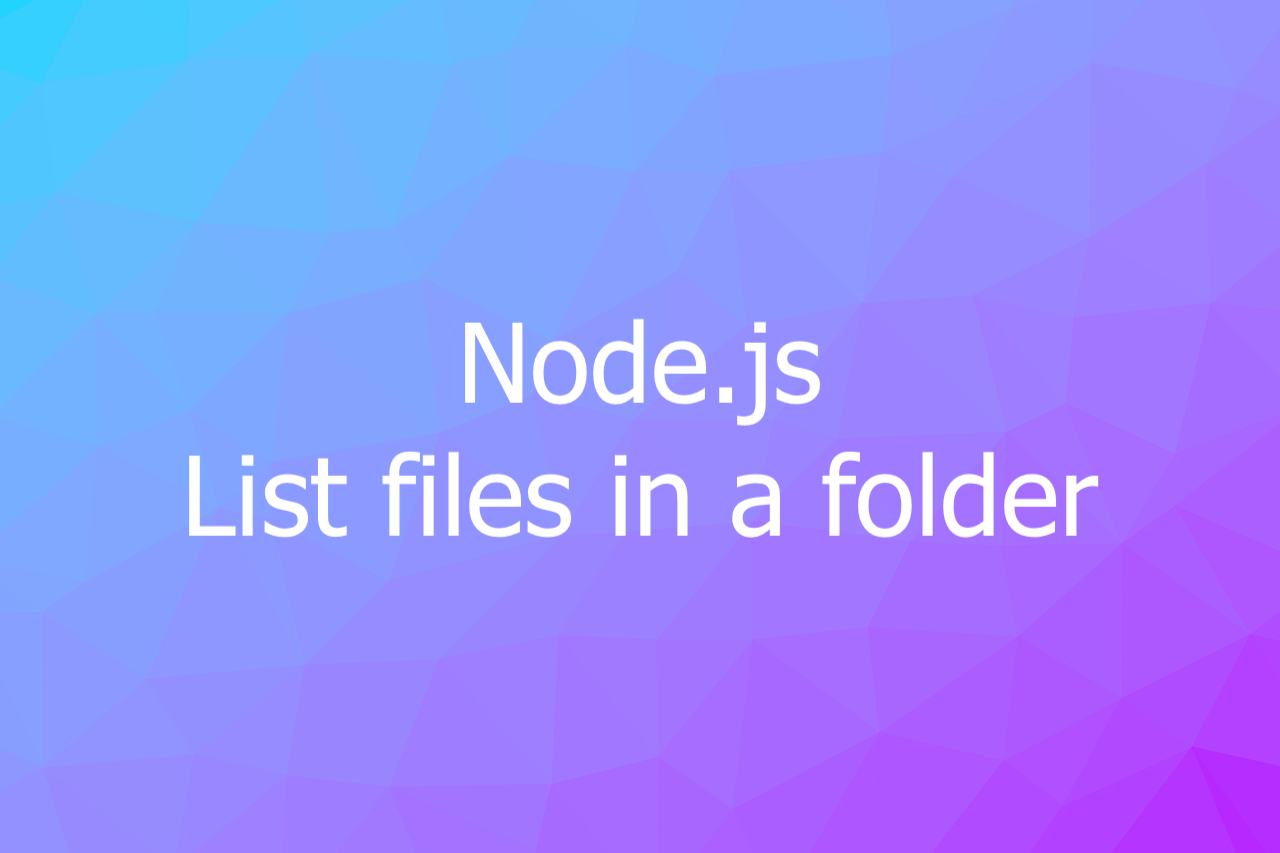
In Node.js, you can asynchronously list all files in a certain directory by using fs.promises.
Example
I have a Node.js project with the file structure as follows:
.
├── files
│ ├── a.jpg
│ ├── b.png
│ ├── c.xml
│ ├── d.csv
│ └── example.txt
├── index.js
└── package.json
Here’s the code in index.js:
import fsPromises from 'fs/promises'
// if you prefer commonJS, use this:
// require fsPromises from 'fs/promises'
const listFiles = async (path) => {
const filenames = await fsPromises.readdir(path);
for (const filename of filenames){
console.log(filename);
}
}
// Test it
listFiles('./files');
The output I get when running the code:
a.jpg
b.png
c.xml
d.csv
example.txt
That’s it. Further reading:
- 2 Ways to Set Default Time Zone in Node.js
- Node.js: How to Compress a File using Gzip format
- Node.js: Using __dirname and __filename with ES Modules
- Tweaking a Node.js Core Module
- Node.js: Use Async Imports (Dynamic Imports) with ES Modules
- 4 Ways to Convert Object into Query String in Node.js
You can also check out our Node.js category page for the latest tutorials and examples.