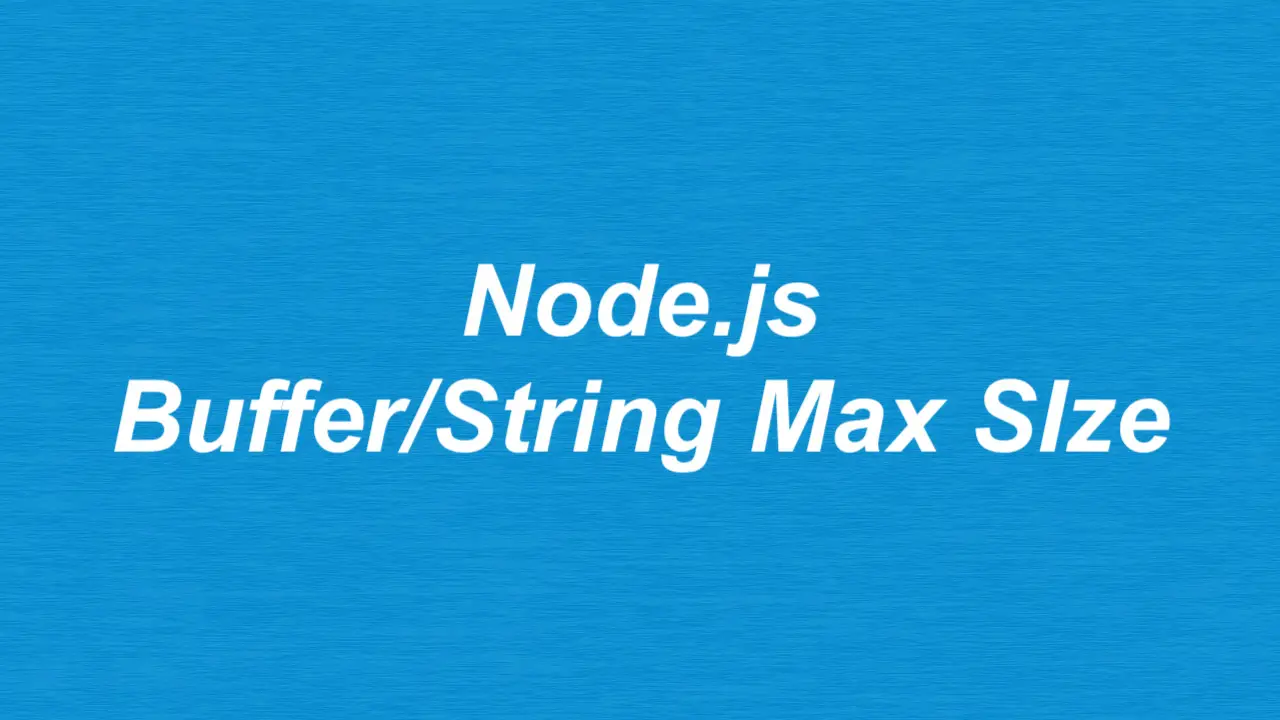
Buffers and strings in Node.js are limited in size. The actual maximum size of a buffer or a string changes across platforms and versions of Node.js. You can check yours as follows:
const buffer = require('buffer');
// Get the results in bytes
console.log(
"The maximum size allowed for a buffer:",
buffer.constants.MAX_LENGTH,
"bytes"
);
console.log(
"The maximum size allowed for a string: ",
buffer.constants.MAX_STRING_LENGTH,
"bytes"
);
// In case you want to get the results in MB
console.log(
"The maximum size allowed for a buffer:",
buffer.constants.MAX_LENGTH / (1024 * 1024),
"MB"
);
console.log(
"The maximum size allowed for a string: ",
buffer.constants.MAX_STRING_LENGTH / (1024 * 1024),
"MB"
);
Here’s my output:
The maximum size allowed for a buffer: 4294967296 bytes
The maximum size allowed for a string: 536870888 bytes
The maximum size allowed for a buffer: 4096 MB
The maximum size allowed for a string: 511.9999771118164 MB
Further reading:
- 4 Ways to Read JSON Files in Node.js
- Node.js: Using __dirname and __filename with ES Modules
- Node.js: Reading and Parsing Excel (XLSX) Files
- Best open-source ORM and ODM libraries for Node.js
- Top 4 best Node.js Open Source Headless CMS
You can also check out our Node.js category page for the latest tutorials and examples.