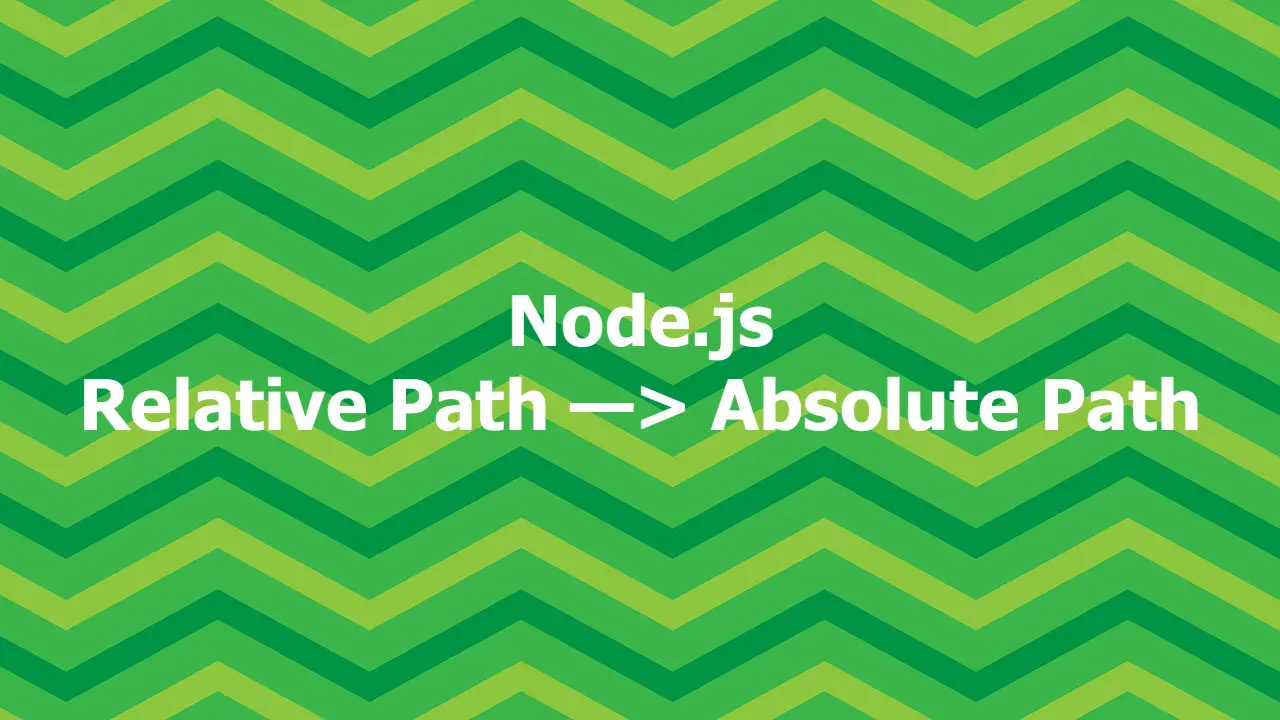
In Node.js, you can convert a relative path to an absolute path simply by using the path module, like this:
import path from 'path'
// if you're using commonJS, use "require" instead of "import":
// const path = require('path')
// a file that locates at the project root
console.log(path.resolve('./test.txt'));
// a file that locates at a subdirectory of the project root
console.log(path.resolve('src/kindacode.jpg'))
// a file that locates at two folders up from the project root
console.log(path.resolve('../../some-thing.txt'));
This is my output on macOS (if you’re using Windows, your result will be more or less different):
/Users/goodman/Desktop/Dev/node/kindacode_js/test.txt
/Users/goodman/Desktop/Dev/node/kindacode_js/src/kindacode.jpg
/Users/goodman/Desktop/Dev/some-thing.txt
That’s it. Further reading:
- Node.js: 2 Ways to Delete All Files in a Folder
- 2 Ways to Set Default Time Zone in Node.js
- 4 Ways to Read JSON Files in Node.js
- Best open-source ORM and ODM libraries for Node.js
- Node.js: How to Use “Import” and “Require” in the Same File
You can also check out our Node.js category page for the latest tutorials and examples.