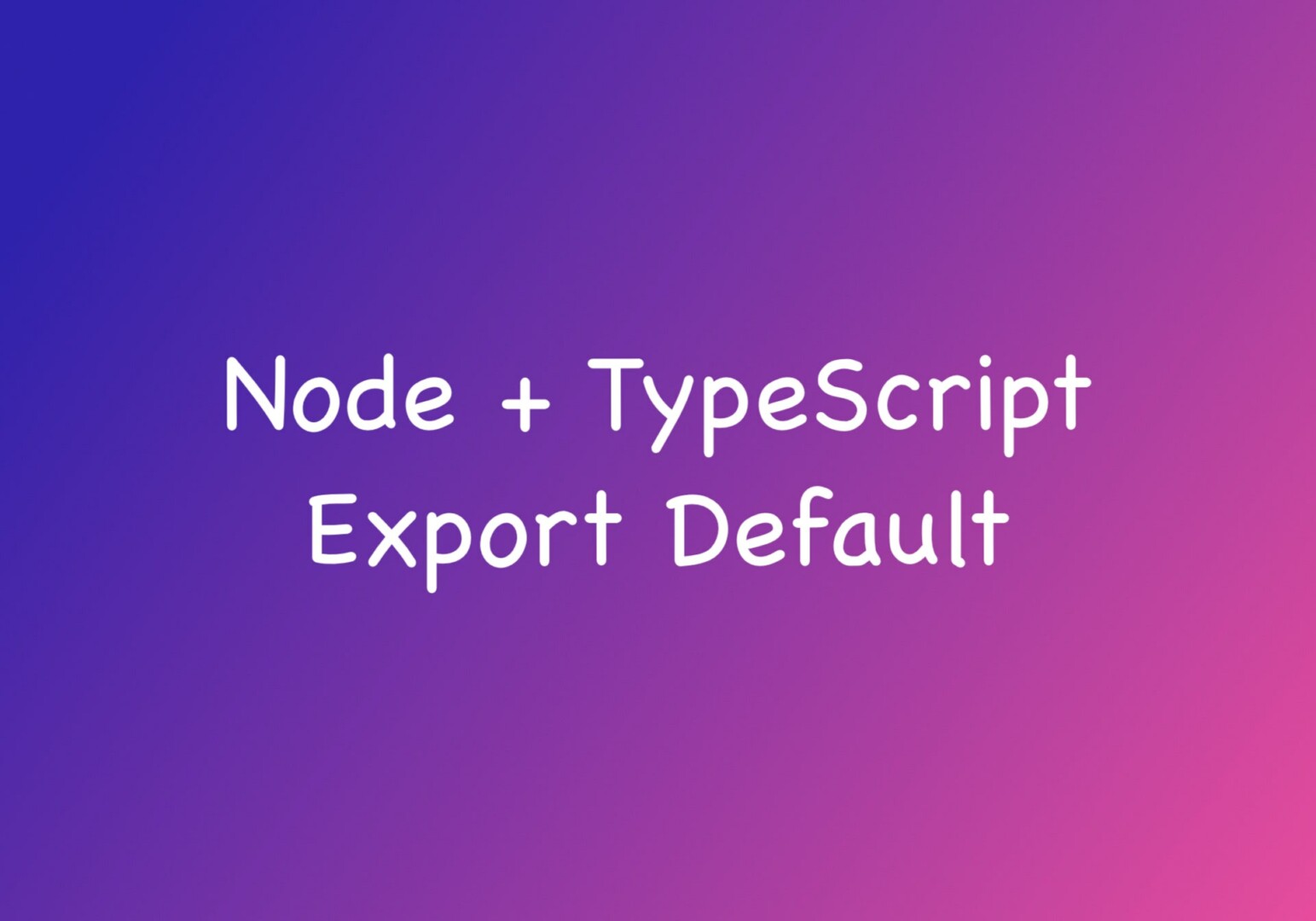
There might be times when you might want to export something based on one or multiple conditions (for example, you want to export configuration information like database, API keys, etc for development environment and production environment). Unfortunately, you cannot put your export statement inside an if/else or switch/case check. To archive the goal, you can do like the example below:
let config;
if (process.env.NODE_ENV === 'development') {
config = {
host: 'localhost',
database: 'test',
username: 'test',
password: '123456'
}
} else {
config = {
/* ... */
}
}
export default config;
The scripts section in package.json:
"scripts": {
"start": "NODE_ENV=development node ./build/index.js",
"dev": "NODE_ENV=development nodemon ./src/index.ts",
"build": "tsc"
},
Further reading:
- Ways to Set Default Time Zone in Node.js
- Using Axios to download images and videos in Node.js
- Node.js: How to Compress a File using Gzip format
- Node.js: Reading and Parsing Excel (XLSX) Files
- How to Easily Upgrade Node.js in macOS
- Node.js: Generate Images from Text Using Canvas
You can also check out our Node.js category page for the latest tutorials and examples.