In React, you can find elements with a certain className using the document.getElementsByClassName() method:
// find the collection of elements with the class name 'my-text'
const collection = document.getElementsByClassName('my-text');
// turn the collection into an array
const myElements = Array.from(collection);
If you only to get the first element in a bunch of elements that have the same className, do like so:
// find the first element with the className of 'container'
const container = document.getElementsByClassName('container')[0];
For more clarity, see the full example below.
Example
This example finds all heading and paragraph elements with the className of my-text, then make them blue. It also seeks the element whose className is container and adds some styles to it.
Screenshot:
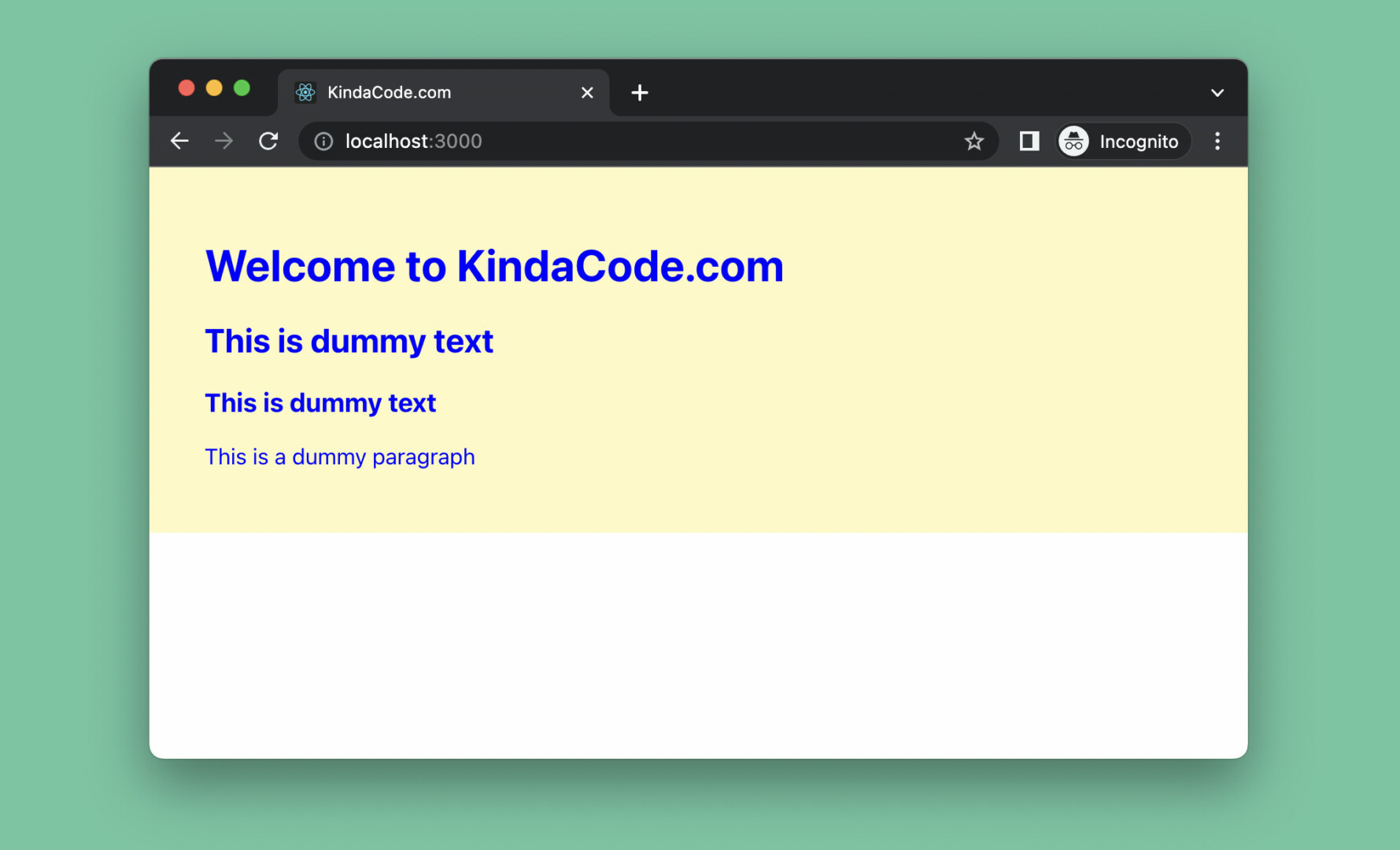
The code (with explanations):
// KindaCode.com
// src/App.js
import { useEffect } from 'react';
function App() {
useEffect(() => {
// find the collection of elements with the class name 'my-text'
const collection = document.getElementsByClassName('my-text');
// turn the collection into an array
const myElements = Array.from(collection);
// loop through the collection of elements
// and style each element
myElements.forEach((element) => {
element.style.color = 'blue';
});
// find the first element with the className of 'container'
const container = document.getElementsByClassName('container')[0];
// then apply some styles to it
container.style.padding = '30px 40px';
container.style.backgroundColor = '#fff9c4';
}, []);
return (
<div className='container'>
<h1 className='my-text'>Welcome to KindaCode.com</h1>
<h2 className='my-text'>This is dummy text</h2>
<h3 className='my-text'>This is dummy text</h3>
<p className='my-text'>This is a dummy paragraph</p>
</div>
);
}
export default App;
That’s it. Further reading:
- React: How to Create a Responsive Navbar from Scratch
- How to get the Width & Height of the Viewport in React
- How to Create a Read More/Less Button in React
- React: Show a Loading Dialog (without any libraries)
- React + TypeScript: Handling onFocus and onBlur events
You can also check our React category page and React Native category page for the latest tutorials and examples.