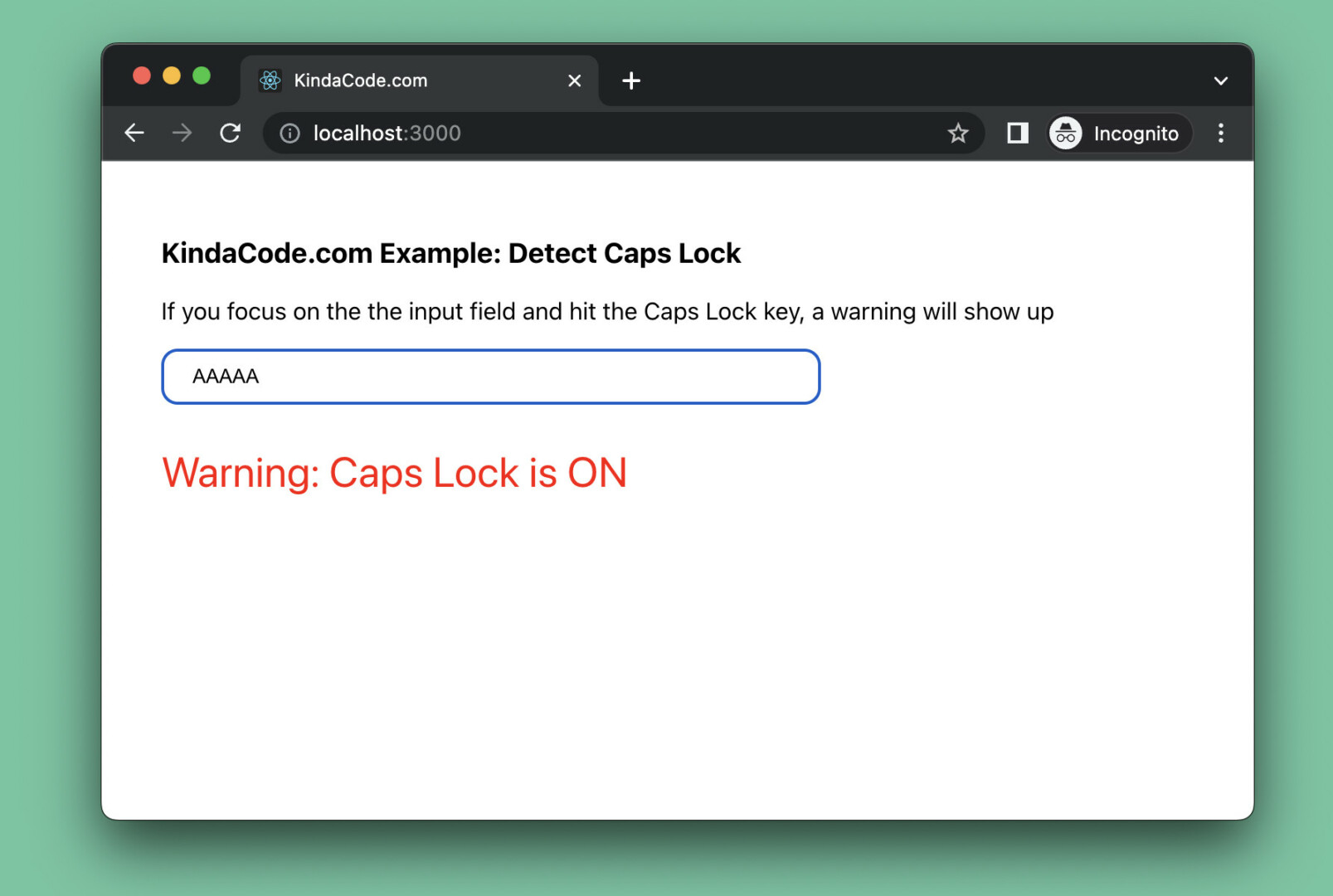
The complete example below shows you how to find out if the caps lock is currently ON when you are typing something into an input field in a React application. This will be useful in many situations, such as when a user types their password, caps lock can cause errors.
Example
Preview:
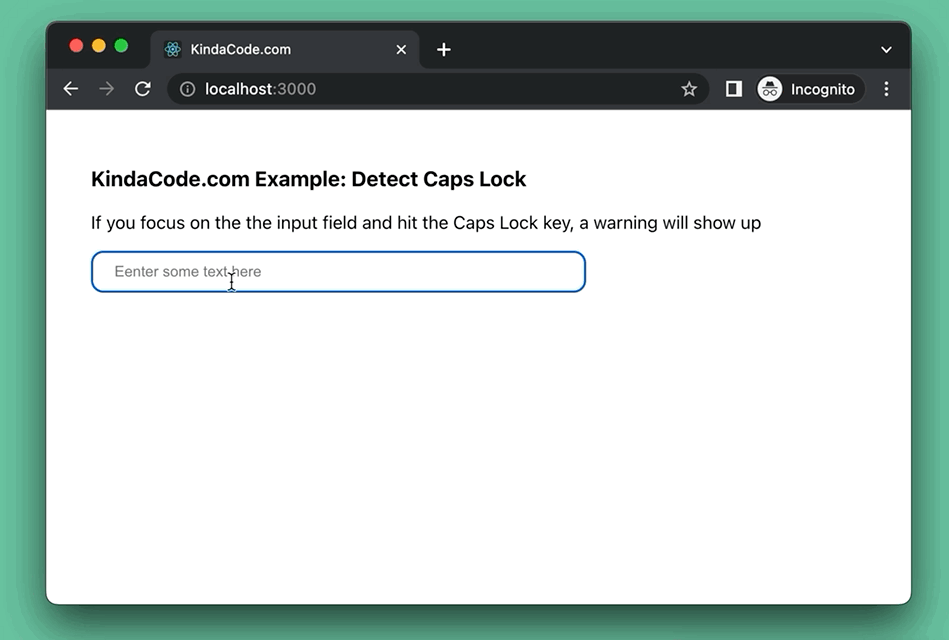
The code in App.js (with explanations):
// KindaCode.com
// src/App.js
import { useState } from 'react';
import './App.css';
function App() {
const [isCapsLockOn, setIsCapsLockOn] = useState(false);
// This function is triggered on the keyup event
const checkCapsLock = (event) => {
if (event.getModifierState('CapsLock')) {
setIsCapsLockOn(true);
} else {
setIsCapsLockOn(false);
}
};
return (
<div className='container'>
<h3>KindaCode.com Example: Detect Caps Lock</h3>
<p>
If you focus on the the input field and hit the Caps Lock key, a warning
will show up
</p>
{/* The input field */}
<input
onKeyUp={checkCapsLock}
type='text'
placeholder='Eenter some text here'
className='input'
/>
{/* Show a warning if caps lock is ON */}
{isCapsLockOn && (
<p className='caps-lock-warning'>Warning: Caps Lock is ON</p>
)}
</div>
);
}
export default App;
The code in App.css:
.container {
margin: 50px 40px;
}
.input {
width: 400px;
padding: 10px 20px;
border: 1px solid #999;
border-radius: 10px;
}
.caps-lock-warning {
color: red;
font-size: 28px;
}
That’s if. Further reading:
- React: How to Create an Image Carousel from Scratch
- React Router: Redirecting with the Navigate component
- React + TypeScript: Handling Keyboard Events
- React + TypeScript: Drag and Drop Example
- React + TypeScript: Multiple Dynamic Checkboxes
- React: Create an Animated Side Navigation from Scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.