Terms of service (aka TOS) are the legal agreements between a website or an app and a person who wants to use that service. Usually, before a user registers an account, there will be a checkbox asking them to agree before they can continue. The example below shows you how to do so in React with the useState hook.
Table of Contents
The Example
App Preview
The simple React project we are going to build contains a checkbox and a button:
- If the checkbox is unchecked, the button will be disabled and can not be clicked.
- If the checkbox is checked, the button will be enabled.
Here’s what you’ll see when running the final project:
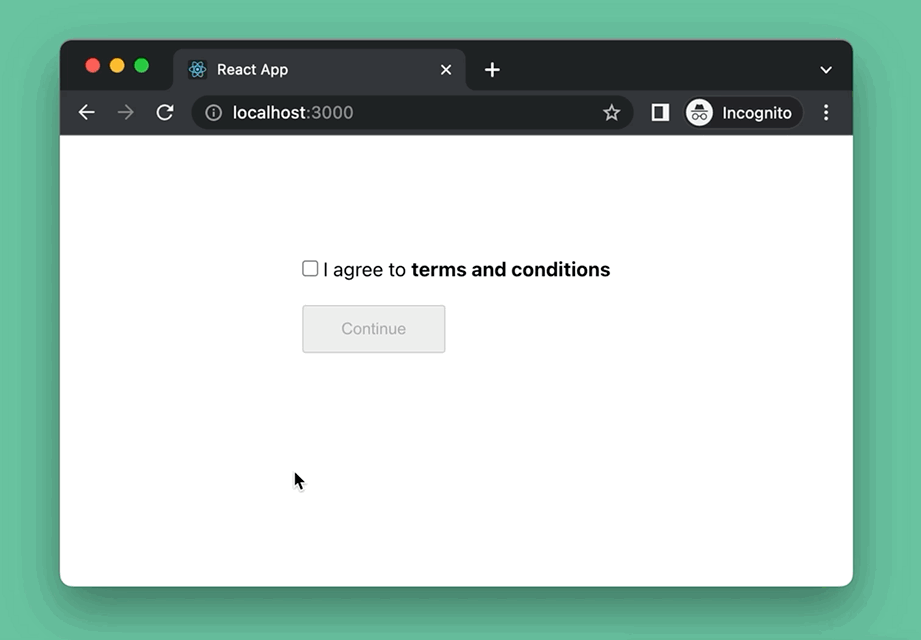
The Code
1. Initialize a new React project:
npx create-react-app kindacode_example
2. Replace the default code in the App.js with the following:
import React, { useState } from 'react';
import './App.css';
const App = () => {
const [agree, setAgree] = useState(false);
const checkboxHandler = () => {
// if agree === true, it will be set to false
// if agree === false, it will be set to true
setAgree(!agree);
// Don't miss the exclamation mark
}
// When the button is clicked
const btnHandler = () => {
alert('The buttion is clickable!');
};
return (
<div className="App">
<div className="container">
<div>
<input type="checkbox" id="agree" onChange={checkboxHandler} />
<label htmlFor="agree"> I agree to <b>terms and conditions</b></label>
</div>
{/* Don't miss the exclamation mark* */}
<button disabled={!agree} className="btn" onClick={btnHandler}>
Continue
</button>
</div>
</div>
);
};
export default App;
3. And here is the App.css:
* {
margin: 0;
padding: 0;
}
.App {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
}
.container {
width: 800;
height: 300;
margin: 100px auto;
}
.btn {
margin-top: 20px;
padding: 10px 30px;
cursor: pointer;
}
Afterword
We’ve walked through a complete example of implementing an “I agree to terms” checkbox. If you’d like to explore more new and interesting things about modern React development, take a look at the following articles:
- Best open-source Admin Dashboard libraries for React
- React + TypeScript: Multiple Dynamic Checkboxes
- React & TypeScript: Using useRef hook example
- React Router Dom: Implement a Not Found (404) Route
- React Router Dom: Scroll To Top on Route Change
- React useReducer hook – Tutorial and Examples
You can also check our React category page and React Native category page for the latest tutorials and examples.