The example below shows you how to make a rounded corners text input in React Native for both Android and iOS.
Screenshot:
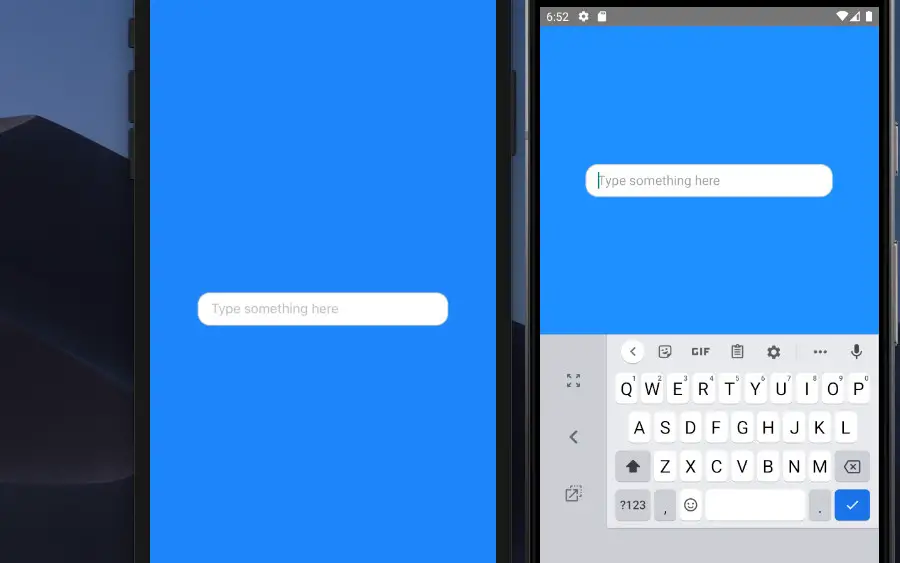
The code
1. Add a TextInput component:
<TextInput style={styles.input} placeholder="Type something here" />
2. Style it:
input: {
width: 300,
height: 40,
backgroundColor: '#fff',
paddingVertical: 10,
paddingHorizontal: 15,
borderColor: '#ccc',
borderWidth: 1,
borderRadius: 15,
fontSize: 16,
},
The complete code:
import React from "react";
import { View, StyleSheet, TextInput } from "react-native";
const App = () => {
return (
<View style={styles.screen}>
<TextInput style={styles.input} placeholder="Type something here" />
</View>
);
};
const styles = StyleSheet.create({
screen: {
width: "100%",
height: "100%",
display: "flex",
justifyContent: "center",
alignItems: "center",
backgroundColor: "#1e90ff",
},
input: {
width: 300,
height: 40,
backgroundColor: "#fff",
paddingVertical: 10,
paddingHorizontal: 15,
borderColor: "#ccc",
borderWidth: 1,
borderRadius: 15,
fontSize: 16,
},
});
export default App;
That’s it. Further reading:
- How to Get the Window Width & Height in React Native
- How to implement tables in React Native
- How to render HTML content in React Native
- React Native: Make a Button with a Loading Indicator inside
- How to create round buttons in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.