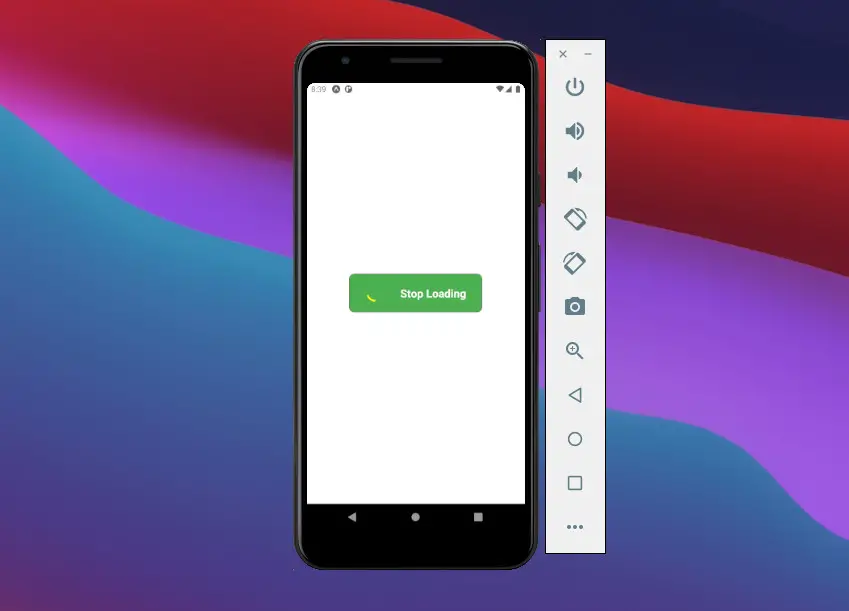
This article walks you through a complete example of implementing a button with a loading indicator inside. We will write code from scratch with only the built-in components of React Native including TouchableOpacity, ActivityIndicator, View, etc. No third-party libraries are required and need to be installed.
Table of Contents
The Example
App Preview
The app we are going to make has a button in the center of the screen. When the button is pressed, its background color will change, and a loading indicator will show up. If you hit the button again, the loading process will stop, and everything will be back to the way it was in the beginning.
A demo is worth more than a thousand words:
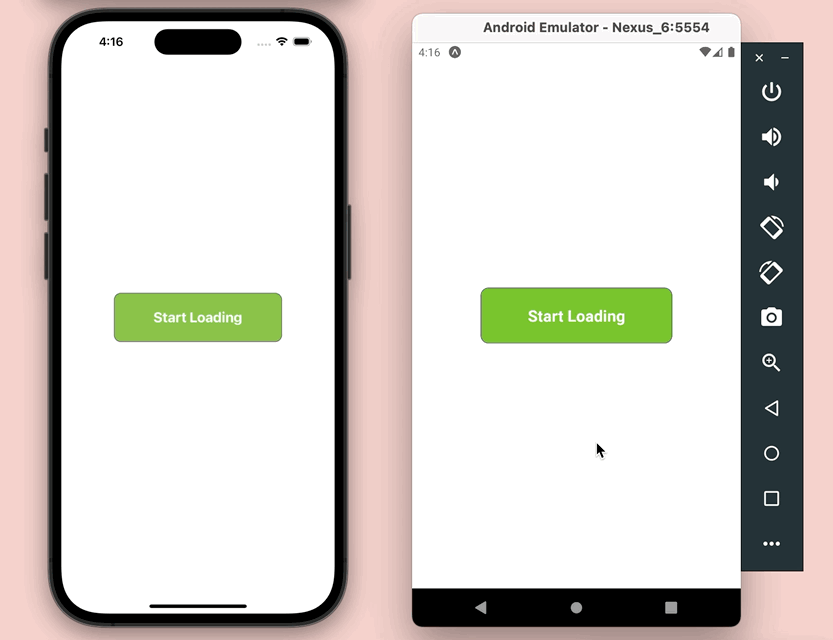
The Complete Code
Replace the unwanted code in your App.js with the following:
// App.js
import React, { useState } from "react";
import {
Text,
StyleSheet,
View,
ActivityIndicator,
TouchableOpacity,
} from "react-native";
export default function App() {
const [isLoading, setIsLoading] = useState(false);
// This function will be triggered when the button is pressed
const toggleLoading = () => {
setIsLoading(!isLoading);
};
return (
<View style={styles.container}>
<TouchableOpacity onPress={toggleLoading}>
<View
style={{
...styles.button,
backgroundColor: isLoading ? "#4caf50" : "#8bc34a",
}}
>
{isLoading && <ActivityIndicator size="large" color="yellow" />}
<Text style={styles.buttonText}>
{isLoading ? "Stop Loading" : "Start Loading"}
</Text>
</View>
</TouchableOpacity>
</View>
);
}
// Kindacode.com
// Just some styles
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: "column",
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center",
},
button: {
display: "flex",
flexDirection: "row",
justifyContent: "space-evenly",
alignItems: "center",
width: 240,
height: 70,
borderWidth: 1,
borderColor: "#666",
borderRadius: 10,
},
buttonText: {
color: "#fff",
fontWeight: "bold",
fontSize: 20
},
});
Final Words
You’ve examined an end-to-end example of creating a button with a loading indicator inside. This thing is very helpful in many real-world scenarios, especially when you want to fetch data from a remote server. If you’d like to explore more new and exciting stuff about modern React Native, take a look at the following articles:
- How to Implement a Picker in React Native
- React Native – How to Update Expo SDK
- Using Image Picker and Camera in React Native (Expo)
- React Native FlatList: Tutorial and Examples
- React Native: How to make rounded corners TextInput
- How to render HTML content in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.