By default, the header title of a React Native app that uses React Navigation 6 is on the left side. To center it, just add this option:
headerTitleAlign: 'center'
Full example:
import React from "react";
import { View, Text, StyleSheet } from "react-native";
import { NavigationContainer } from "@react-navigation/native";
import { createNativeStackNavigator } from "@react-navigation/native-stack";
const MainStack = createNativeStackNavigator();
// implement Home Screen
const HomeScreen = (props) => {
return (
<View style={styles.screen}>
<Text>Home Screen</Text>
</View>
);
};
function App() {
return (
<NavigationContainer>
<MainStack.Navigator>
<MainStack.Screen
name="home"
component={HomeScreen}
options={{
title: "Home Title",
// Center the header title on Android
headerTitleAlign: "center",
}}
/>
</MainStack.Navigator>
</NavigationContainer>
);
}
// Kindacode.com
/// Just some styles
const styles = StyleSheet.create({
screen: {
flex: 1,
justifyContent: "center",
alignItems: "center",
},
});
export default App;
Screenshot:
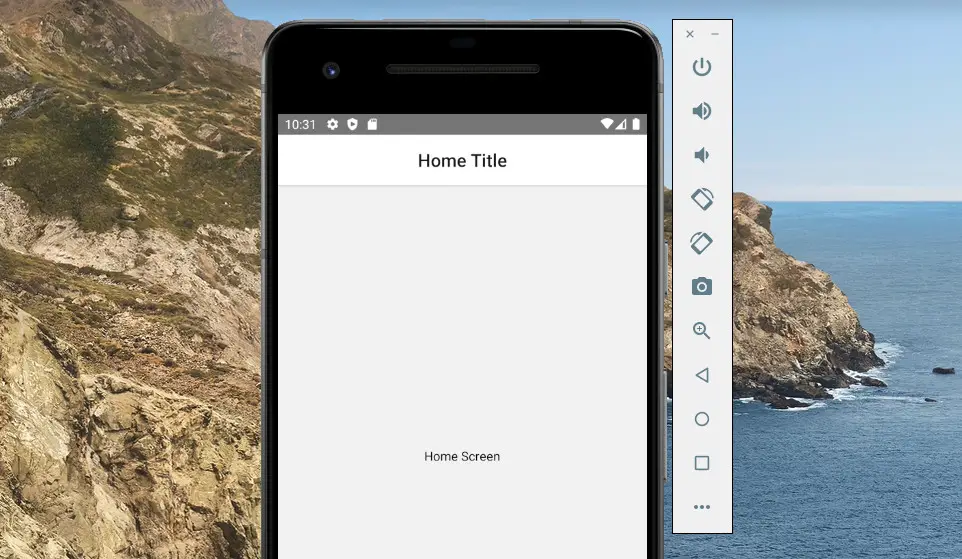
Further reading:
- React Native: How to make rounded corners TextInput
- React Navigation: useRoute hook example
- React Navigation: Dynamic header title (with hooks)
- React Native: Make a Button with a Loading Indicator inside
- Implementing a Date Time picker in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.