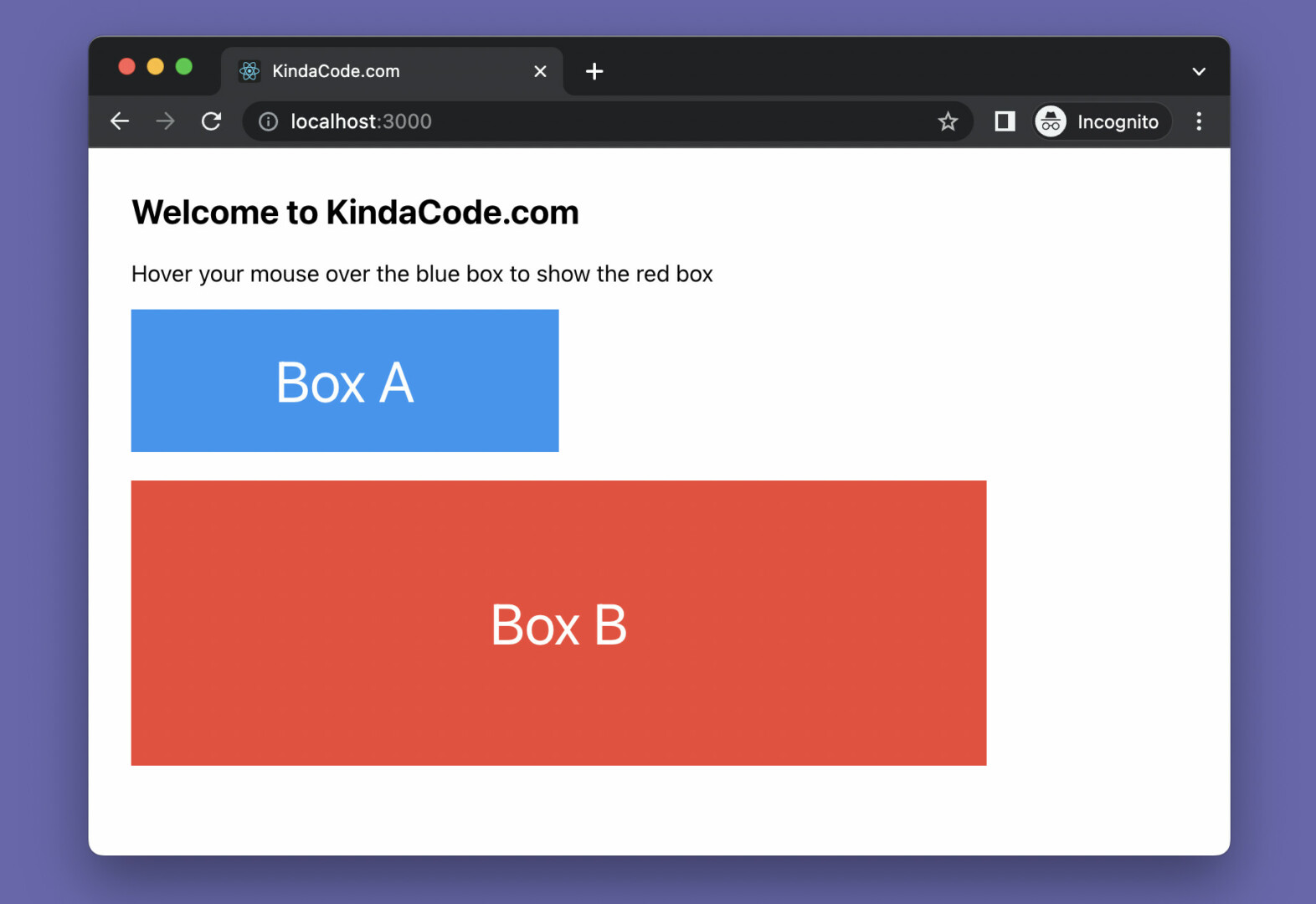
Let’s say we’re developing a React app, and we want to programmatically show a red box named box B when the mouse cursor is hovering over another box called box A. To achieve the goal, we can make use of the onMouseOver and onMouseOut events in React:
- Use a state variable to determine whether box B is shown or not. The default value is false
- Add the onMouseOver and onMouseOut props to box A
- When the onMouseOver event is fired (that means the user is currently hovering over box A), set the state variable to true
- When the onMouseOut event is fired, set the state variable to false
For more clarity, see the complete example below.
App Preview:
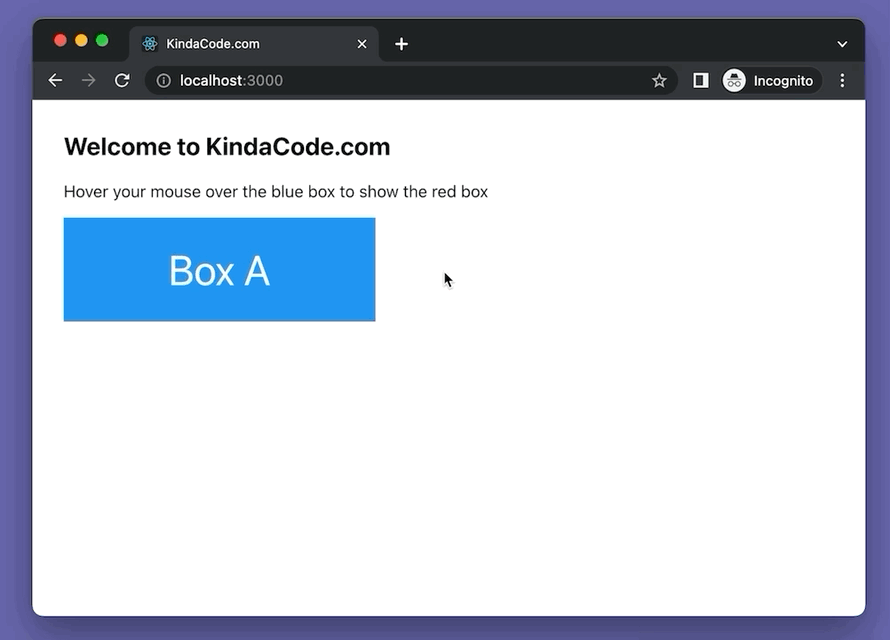
The full code (with explanations):
// KindaCode.com
// src/App.js
import { useState } from 'react';
function App() {
// this state determines whether box B is shown or not
const [isShown, setIsShown] = useState(false);
// this function is called when the mouse hovers over box A
const handleMouseOver = () => {
setIsShown(true);
}
// this function is called when the mouse out box A
const handleMouseOut = () => {
setIsShown(false);
}
return (
<div style={styles.container}>
<h2>Welcome to KindaCode.com</h2>
<p>Hover your mouse over the blue box to show the red box</p>
<div onMouseOver={handleMouseOver} onMouseOut={handleMouseOut} style={styles.boxA}>Box A</div>
{/* Conditionally show/hide box B */}
{isShown && <div style={styles.boxB}>Box B</div>}
</div>
);
}
// Styles
const styles = {
container: {
padding: '10px 30px'
},
// Box A styles
boxA: {
width: 300,
height: 100,
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
background: '#2196f3',
color: '#fff',
fontSize: '40px'
},
// Box B styles
boxB: {
marginTop: 20,
width: 600,
height: 200,
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
background: '#f44336',
color: '#fff',
fontSize: '40px'
},
};
export default App;
Further reading:
- React: Load and Display Data from Local JSON Files
- React + TypeScript: onMouseOver & onMouseOut events
- React + TypeScript: Multiple Dynamic Checkboxes
- How to Use Tailwind CSS in React
- React: How to Create an Image Carousel from Scratch
- React: How to Create a Reorderable List from Scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.