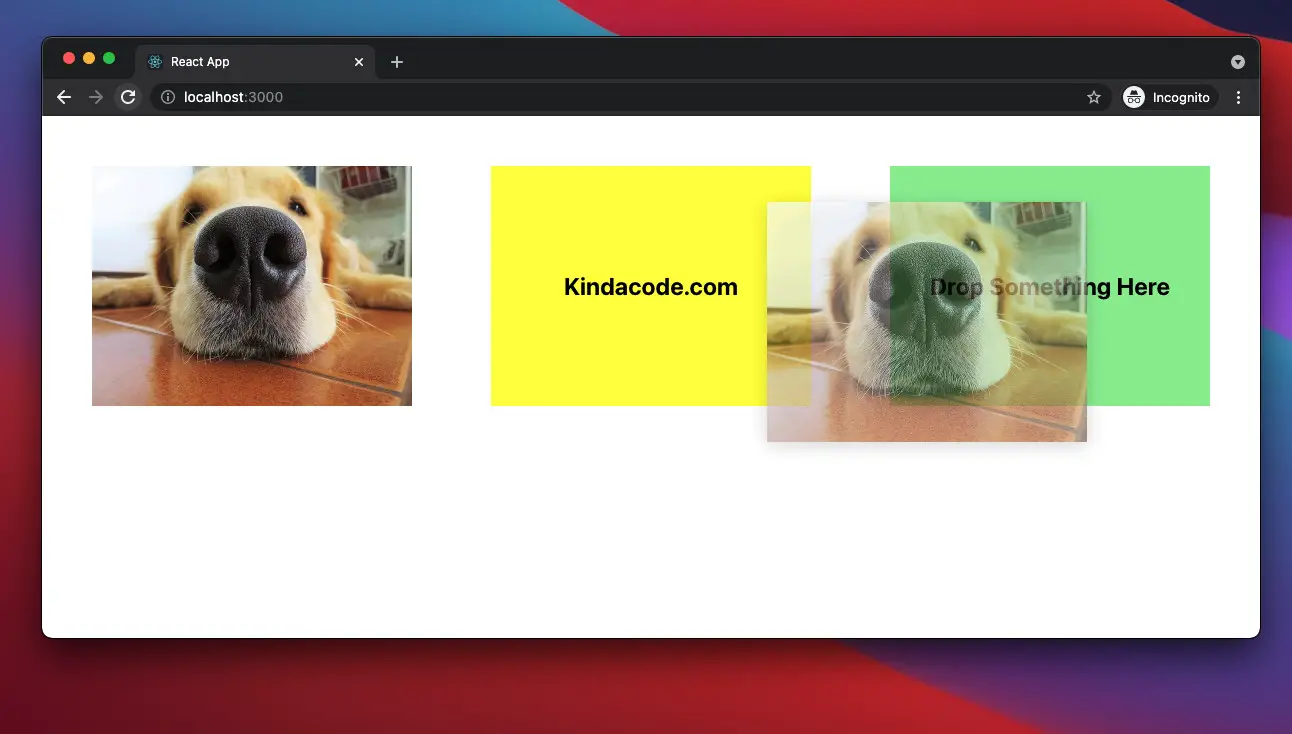
This article walks you through a complete example of implementing drag and drop in a React application that uses TypeScript. We are going to write code from scratch and not using any third-party packages.
Table of Contents
Example
In this example, we can drag the cute dog image (Image source: Pixabay) in the first box or drag the text in the second box (the yellow one) and drop it into the third box (the green).
Preview
The Code
1. Create a new React project by running:
npx create-react-app drag_drop_example --template typescript
2. Replace the default code in your src/App.jsx with the following:
// App.tsx
// Kindacode.com
import React, { useState } from "react";
import "./App.css";
const PHOTO_URL =
"https://www.kindacode.com/wp-content/uploads/2021/06/cute-dog.jpeg";
const App = () => {
// The content of the target box
const [content, setContent] = useState<string>("Drop Something Here");
// This function will be triggered when you start dragging
const dragStartHandler = (
event: React.DragEvent<HTMLDivElement>,
data: string
) => {
event.dataTransfer.setData("text", data);
};
// This function will be triggered when dropping
const dropHandler = (event: React.DragEvent<HTMLDivElement>) => {
event.preventDefault();
const data = event.dataTransfer.getData("text");
setContent(data);
};
// This makes the third box become droppable
const allowDrop = (event: React.DragEvent<HTMLDivElement>) => {
event.preventDefault();
};
return (
<div className="container">
<div
className="box1"
onDragStart={(event) => dragStartHandler(event, PHOTO_URL)}
draggable={true}
>
<img src={PHOTO_URL} alt="Cute Dog" />
</div>
<div
className="box2"
onDragStart={(event) => dragStartHandler(event, "Kindacode.com")}
draggable={true}
>
<h2>Kindacode.com</h2>
</div>
<div className="box3" onDragOver={allowDrop} onDrop={dropHandler}>
{content.endsWith(".jpeg") ? <img src={content} /> : <h2>{content}</h2>}
</div>
</div>
);
};
export default App;
3. Remove all the unwanted code in your src/App.css and add this:
/*
App.css
Kindacode.com
*/
.container {
padding: 50px;
display: flex;
justify-content: space-between;
}
.box1 {
width: 320px;
height: 240px;
}
img {
max-width: 320px;
}
.box2 {
width: 320px;
height: 240px;
background: yellow;
display: flex;
justify-content: center;
align-items: center;
}
.box3 {
width: 320px;
height: 240px;
background: lightgreen;
display: flex;
justify-content: center;
align-items: center;
}
Conclusion
We’ve gone over a full example of dragging and dropping in React and TypeScript. If you would like to learn more about modern front-end development, take a look at the following articles:
- React & TypeScript: Using useRef hook example
- 5 best open-source WYSIWYG editors for React
- React + TypeScript: Handling form onSubmit event
- React + TypeScript: Making a Reading Progress Indicator
- React + TypeScript: Working with Props and Types of Props
- React + TypeScript: Handle onCopy, onCut, and onPaste events
You can also check our React category page and React Native category page for the latest tutorials and examples.