The simple example below shows you how to get the value from an input field in React.
App preview
The sample app we are going to build has a text input. When the user types something into this input, the result will be immediately reflected on the screen:
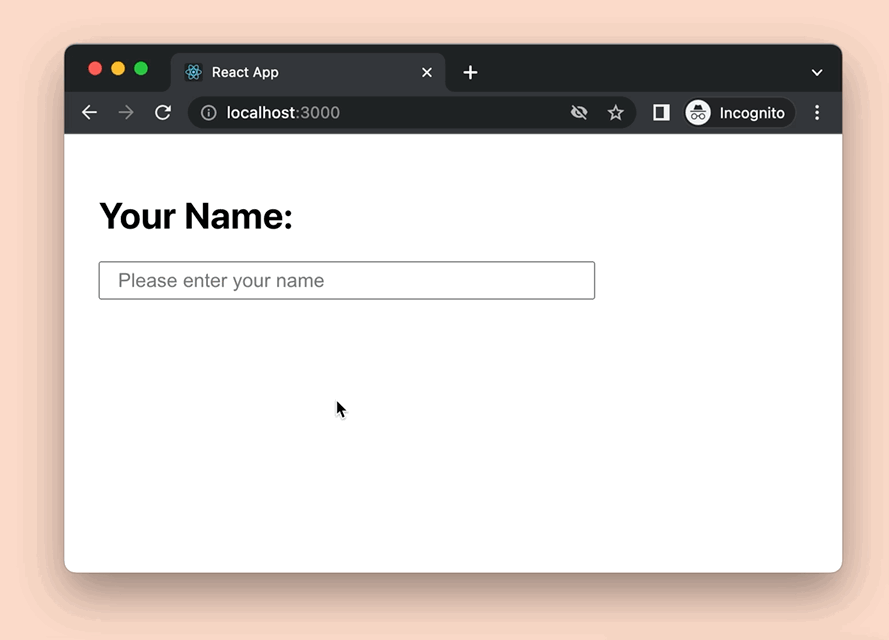
The code
Create a brand new React app, delete everything in the App.js file, and add the code below:
import React, { useState } from 'react';
const App = () => {
const [name, setName] = useState('');
const updateName = (event) => {
setName(event.target.value);
};
return (
<div style={{ padding: 30 }}>
<h1>Your Name: {name}</h1>
<form>
<input
type='text'
value={name}
onChange={updateName}
placeholder='Please enter your name'
style={{ padding: '5px 15px', width: 400, fontSize: 17 }}
/>
</form>
</div>
);
};
export default App;
Try your work by running:
npm start
That’s it.
Further reading:
- How to fetch data from APIs with Axios and Hooks in React
- Top 4 best React form validation libraries
- React: Using inline styles with the calc() function
- React + TypeScript: Handling onScroll event
- React + TypeScript: Handling onFocus and onBlur events
- React + TypeScript: Making a Custom Context Menu
You can also check our React category page and React Native category page for the latest tutorials and examples.