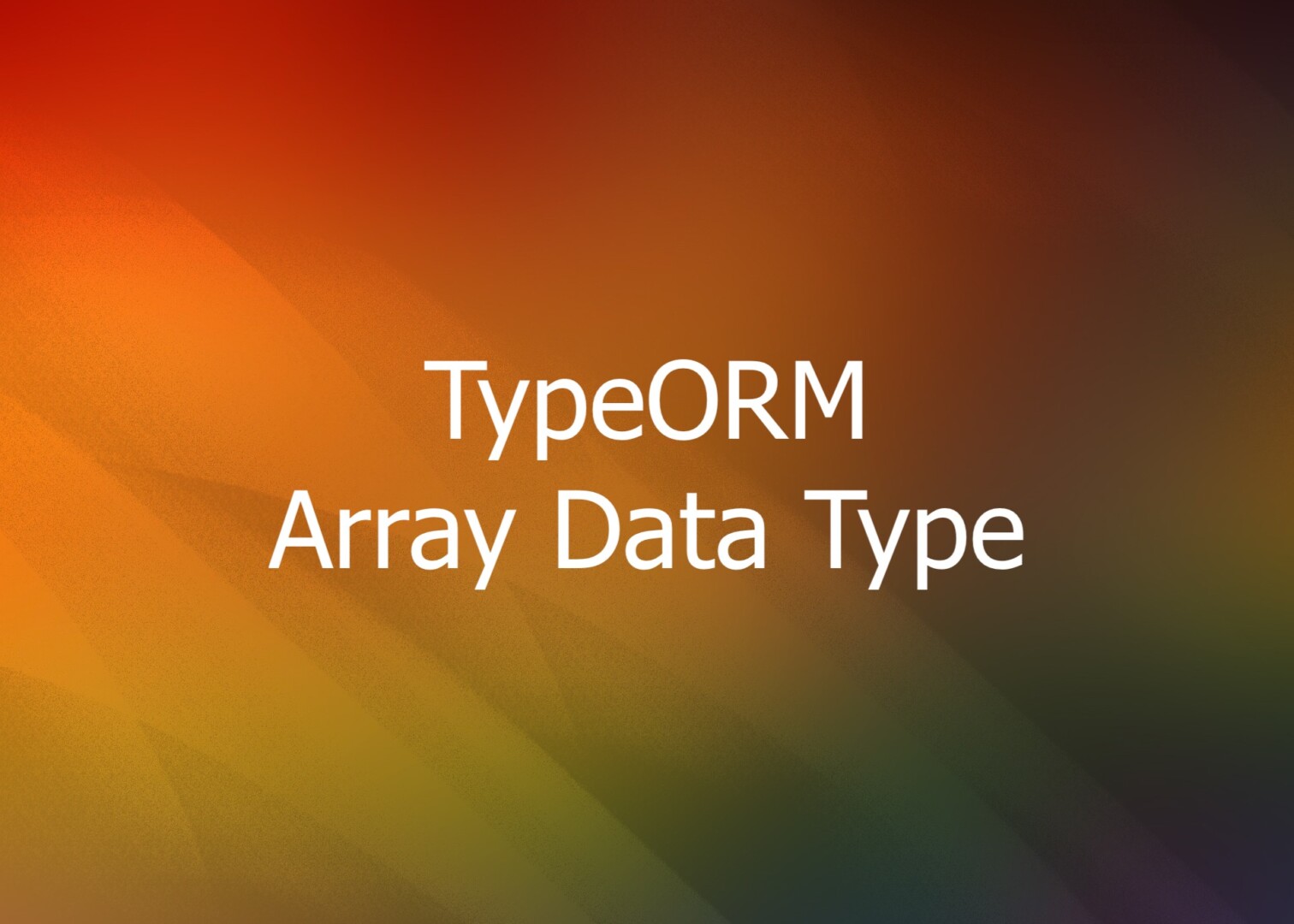
When using TypeORM, it’s possible to define columns with the array data type.
If you want to add a column that stores arrays of numbers, you can define it like so:
@Column('integer', {
array: true, // this is supported by postgreSQL only
nullable: true,
})
sizes: number[];
In case you need a column that stores arrays of strings, you can define it like this:
@Column('simple-array', { nullable: true })
colors: string[];
It is essential to be aware that you MUST NOT have any comma in any element of your array.
The example below creates a table named products whose some columns’ types are array:
// Product entity
import {
Entity,
PrimaryGeneratedColumn,
Column,
CreateDateColumn,
} from 'typeorm';
@Entity({name: 'products'})
export class Product {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
// array of numbers
@Column('integer', {
array: true, // this is supported by postgres only
nullable: true,
})
sizes: number[];
// array of strings
@Column('simple-array', { nullable: true })
colors: string[];
@Column({ type: 'simple-array', nullable: true })
materials: string[];
@CreateDateColumn()
createdAt: Date;
}
And here’s how we save a new record:
const productRepository = dataSource.getRepository(Product);
const product = new Product();
product.name = 'KindaCode.com';
product.sizes = [1, 2, 3];
product.colors = ['red', 'blue', 'green'];
product.materials = ['wood', 'metal', 'plastic'];
await productRepository.save(product);
When viewing the data you’ve added to your database, you’ll see something like this:
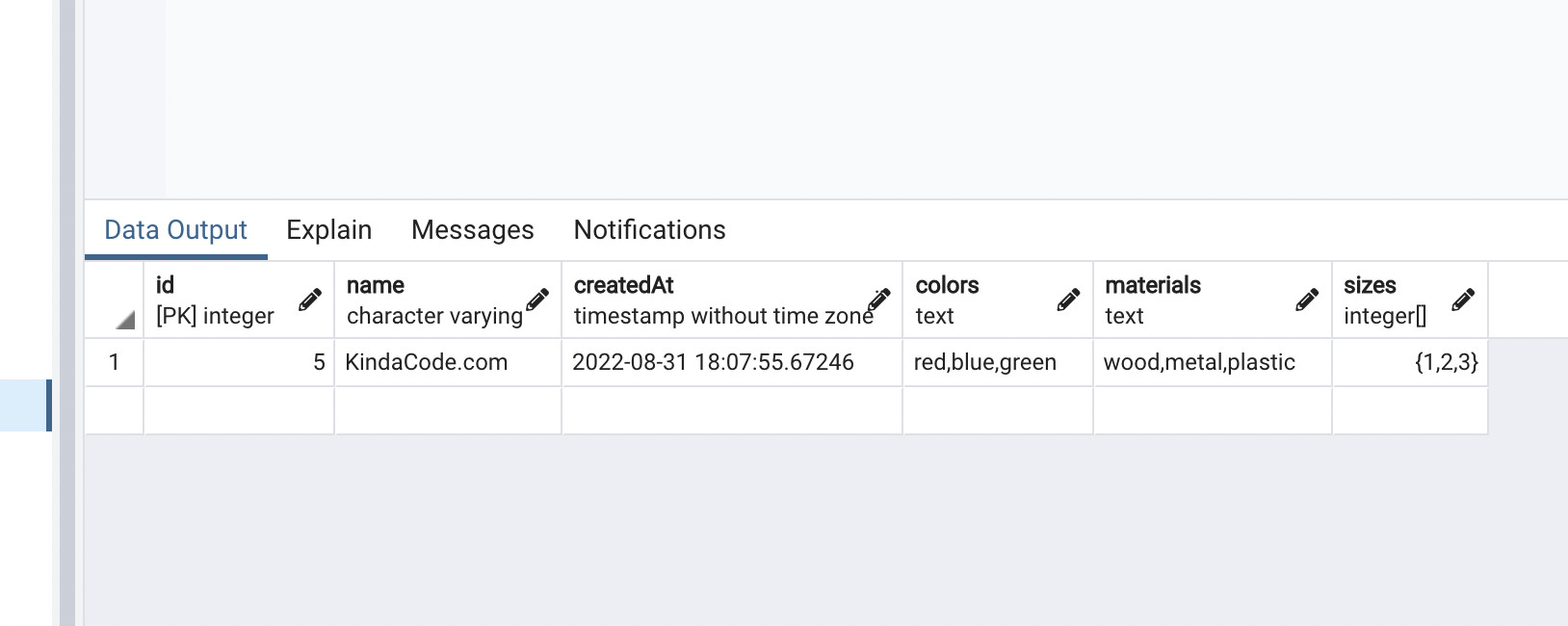
Further reading:
- Pagination in TypeORM (Find Options & QueryBuilder)
- TypeORM: Entity with Decimal Data Type
- TypeORM: Selecting Rows Between 2 Dates
- TypeORM: Selecting Rows with Null Values
- TypeORM: AND & OR operators
- TypeORM: Adding created_at and updated_at columns
You can also check out our database topic page for the latest tutorials and examples.