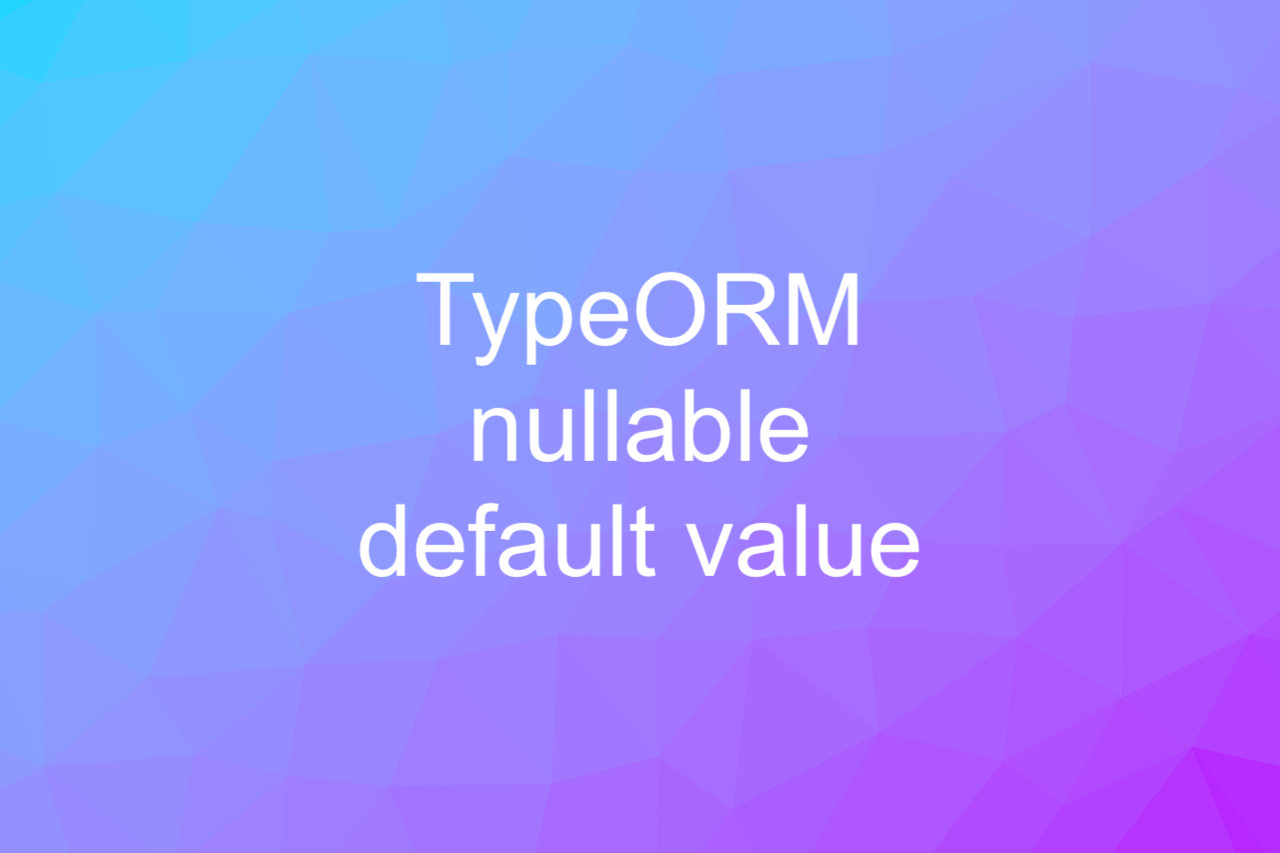
By default, every field (column) of a TypeORM entity is NOT NULL. You can make a specific field become nullable by setting the nullable option to true, like this:
// book.entity.ts
import {
Entity,
PrimaryGeneratedColumn,
Column,
} from 'typeorm'
@Entity()
export class Book {
@PrimaryGeneratedColumn()
id: number;
@Column()
title: string;
// This column is nullable
@Column({ nullable: true })
auther: string;
}
In case you want to add the column’s DEFAULT value:
// book.entity.ts
import {
Entity,
PrimaryGeneratedColumn,
Column,
} from 'typeorm'
@Entity()
export class Book {
@PrimaryGeneratedColumn()
id: number;
@Column()
title: string;
// This column is nullable
@Column({ nullable: true })
auther: string;
// The default price = 0 means a book is free
@Column({default: 0})
price: number;
}
You can learn more about TypeORM in the official docs (typeorm.io).
Further reading:
- Using ENUM Type in TypeORM
- Unsigned (non-negative) Integer in PostgreSQL
- TypeORM: Property ‘id’ has no initializer and is not definitely assigned in the constructor
- TypeORM: Adding created_at and updated_at columns
- 2 Ways to View the Structure of a Table in PostgreSQL
You can also check out our database topic page for the latest tutorials and examples.