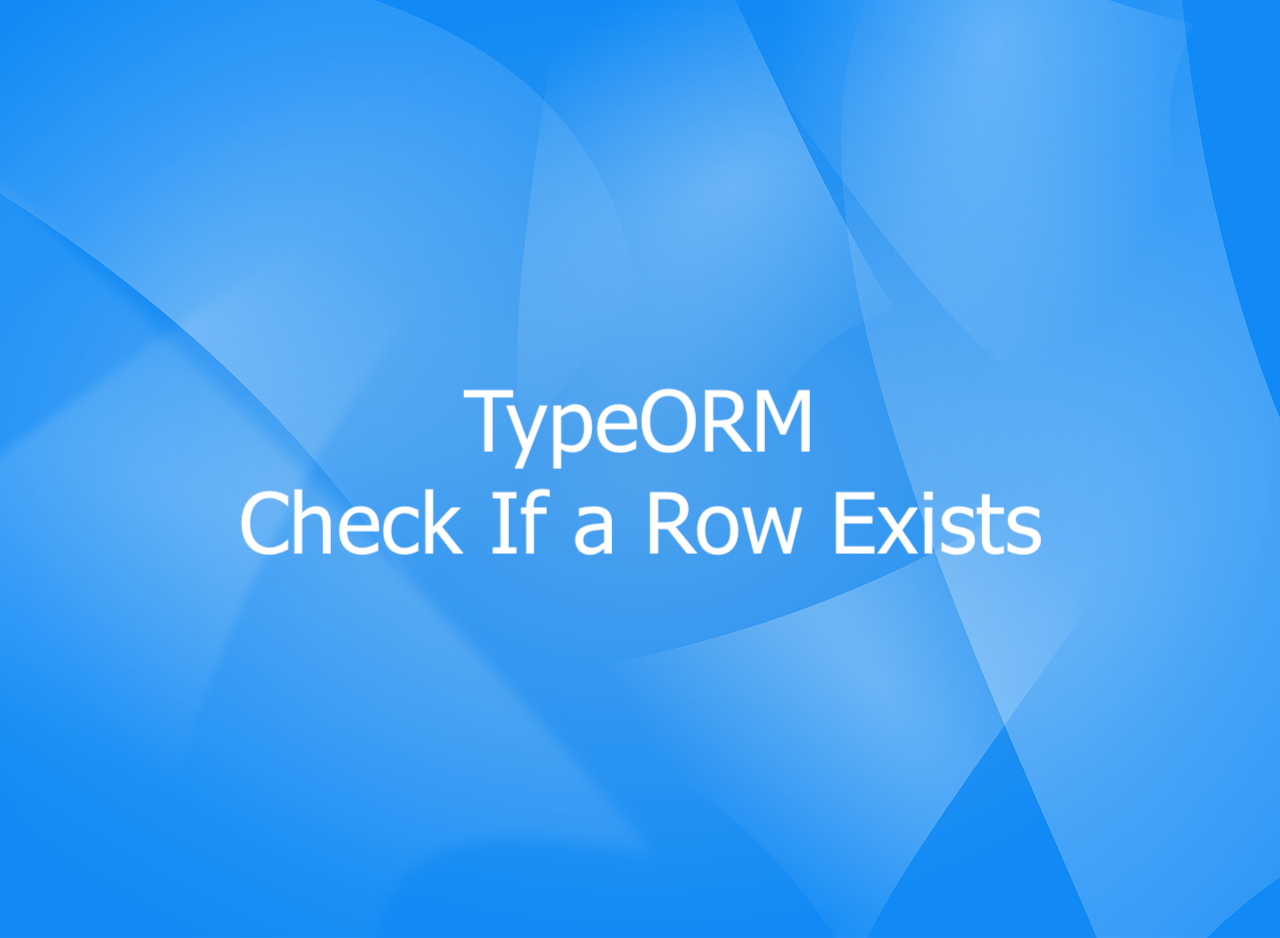
The 2 examples below demonstrate how to check if a record exists or not by using TypeORM findOne() method and query builder, respectively.
Using the findOne() method
Suppose we need to find a product named KatherineCode.com, we can do this:
const productRepository = myDataSource.getRepository(Product);
const product = await productRepository.findOne({
where: {
name: 'KindaCode.com'
}
})
if(product) {
console.log(product);
} else {
console.log('Product not found');
}
Using query builder
In case you prefer using query builder to the find options, you can use this code:
const productRepository = myDataSource.getRepository(Product);
const product = await productRepository
.createQueryBuilder('product')
.where('product.name = :productName', { productName: 'KindaCode.com' })
.getOne();
if (product) {
console.log(product);
} else {
console.log('Product not found');
}
That’s it. Further reading:
- How to Store JSON Object with TypeORM
- TypeORM: Add Columns with Array Data Type
- Pagination in TypeORM (Find Options & QueryBuilder)
- TypeORM: AND & OR operators
- Best open-source ORM and ODM libraries for Node.js
You can also check out our Javascript category page, TypeScript category page, Node.js category page, and React category page for the latest tutorials and examples.