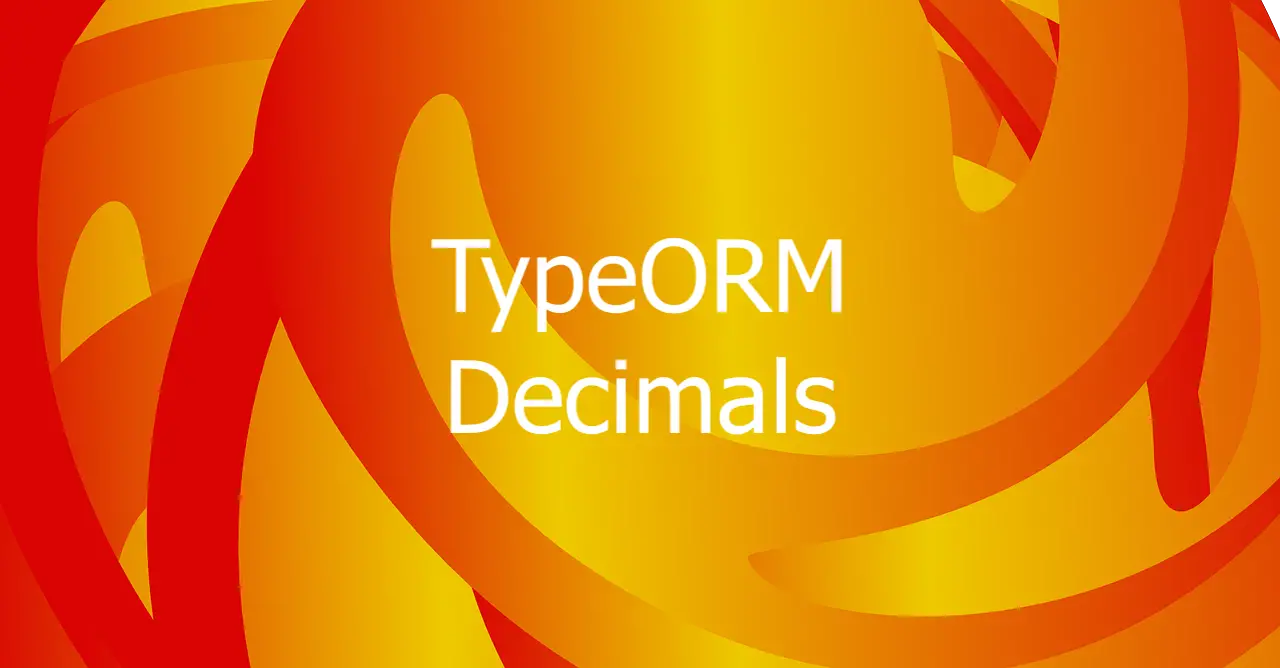
In TypeORM, you can add a column with decimal data type like this:
@Column({type: "decimal", precision: 10, scale: 2, default: 0})
price: number;
Where:
- precision: The number of digits in the decimal
- scale: The number of digits to the right of the decimal point
Full example:
// Product entity
import { Entity, PrimaryGeneratedColumn, Column, CreateDateColumn } from 'typeorm'
@Entity()
export class Product {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column({type: "decimal", precision: 10, scale: 2, default: 0})
price: number;
@CreateDateColumn()
createdAt: Date;
}
Further reading:
- TypeORM: Selecting Rows Between 2 Dates
- TypeORM: AND & OR operators
- TypeORM: How to store BIGINT correctly
- TypeORM: 2 Ways to Exclude a Column from being Selected
- Using ENUM Type in TypeORM
- Best open-source ORM and ODM libraries for Node.js
You can also check out our database topic page for the latest tutorials and examples.