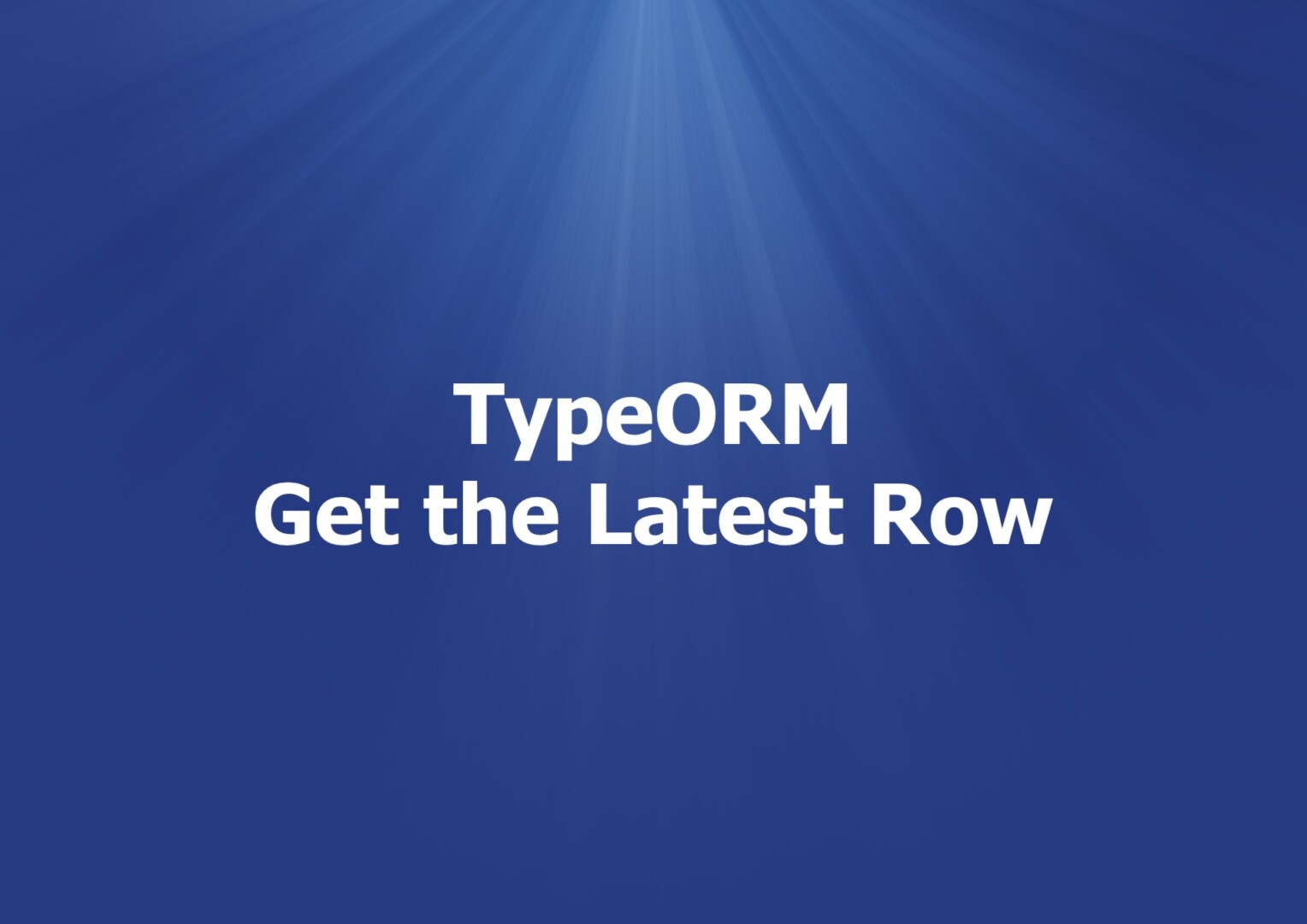
This short article walks you through 2 examples of selecting the latest record from a table using TypeORM. The first example uses the findOne() method, while the second one uses a query builder.
Using the findOne() method
This code snippet demonstrates how to get the latest blog post from the database:
const postRepository = myDataSource.getRepository(Post);
const latestPost = await postRepository.findOne({
where: {
/* You can leave this empty or add your own conditions */
},
order: { id: 'DESC' },
});
console.log(latestPost);
Using a query builder
This gives you the same result as the preceding example:
const postRepository = myDataSource.getRepository(Post);
const latestPost = await postRepository
.createQueryBuilder('post')
.select()
.orderBy('post.id', 'DESC')
.getOne();
console.log(latestPost);
Further reading:
- TypeORM: Using LIKE Operator (2 Examples)
- TypeORM Upsert: Update If Exists, Create If Not Exists
- TypeORM: AND & OR operators
- TypeORM: Check Whether a Row Exists or Not
- TypeORM: Adding Fields with Nullable/Default Data
You can also check out our Javascript category page, TypeScript category page, Node.js category page, and React category page for the latest tutorials and examples.