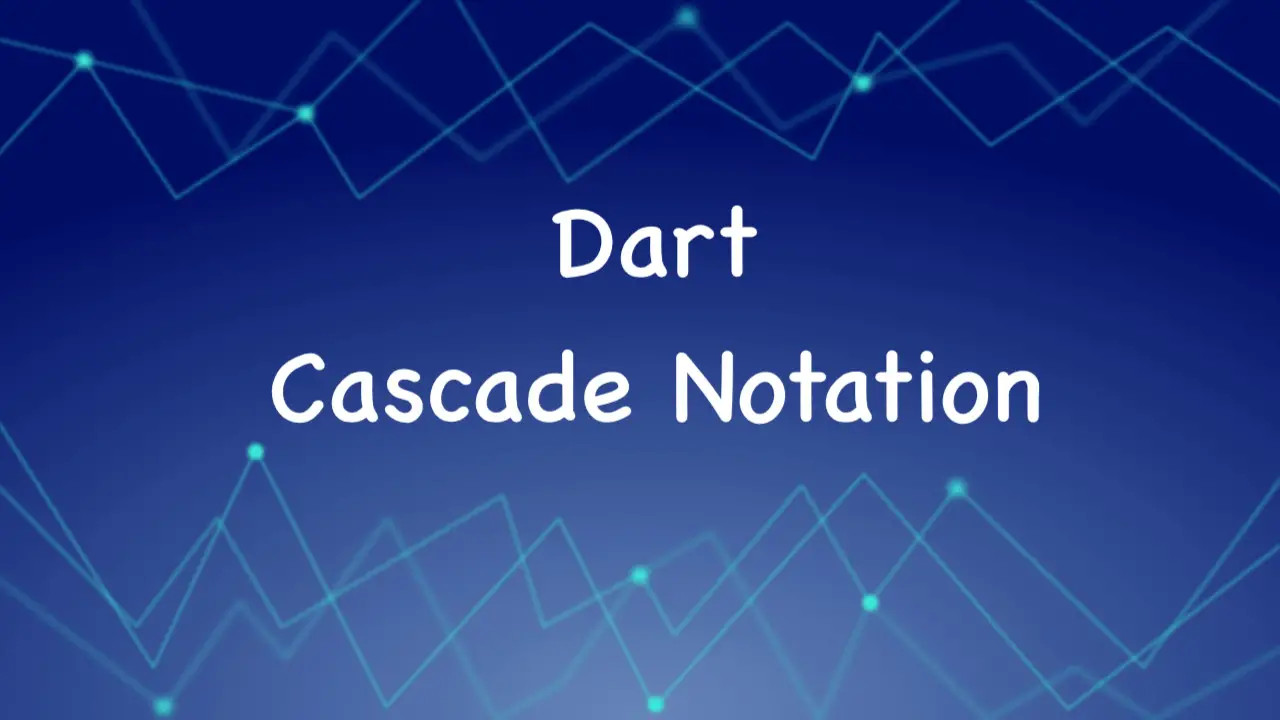
This short post walks you through a few examples of using the cascade notation (.., ?…) in Dart.
What Is The Point?
In Dart, cascades let you execute a sequence of operations on the same object. In other words, cascades allow you to perform a chain of methods without the need for temporary variables. In general, they will look like this:
myObject..method1()..method2()..method3();
myList?..method1()..method2()..method3()..method4();
For more clarity, see the examples below.
Example 1: Cascades and a List
The two code snippets below do the same thing.
1. Using cascades:
// KindaCode.com
void main() {
List myList = [1, 2, 3];
myList
..clear()
..add(4)
..add(5)
..add(6);
print(myList);
}
// Output: [4, 5, 6]
2. The code snippet below rewrites the preceding example but not using cascade:
void main() {
List myList = [1, 2, 3];
myList.clear();
myList.add(4);
myList.add(5);
myList.add(6);
print(myList);
}
// Output: [4, 5, 6]
Example 2: Cascades and a Class
The code:
// KindaCode.com
// Define a class called "Person" with "name" and "age" properties
class Person {
String? name;
int? age;
void setName(String name) {
this.name = name;
}
void setAge(int age) {
this.age = age;
}
void printInfo() {
print("User name: ${name ?? 'Unknown'}");
print("User age: ${age ?? 'Not provided'}");
}
}
void main() {
Person()
..setName('Kindacode.com')
..setAge(4)
..printInfo();
final instance = Person();
instance
..setName('John Doe')
..setAge(99)
..printInfo();
}
Output:
// Person 1
User name: Kindacode.com
User age: 4
// Person 2
User name: John Doe
User age: 99
Conclusion
We’ve gone through a couple of examples of using cascades in Dart. At this point, you should have a better understanding and get a comfortable sense of reading and writing concise and compact code with this syntax. If you’d like to explore more new and interesting features in Dart and Flutter, take a look at the following posts:
- Dart: Convert Timestamp to DateTime and vice versa
- Dart & Flutter: Get the Index of a Specific Element in a List
- 2 ways to remove duplicate items from a list in Dart
- Dart regular expressions to check people’s names
- Flutter: ListView Pagination (Load More) example
- Flutter & SQLite: CRUD Example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.