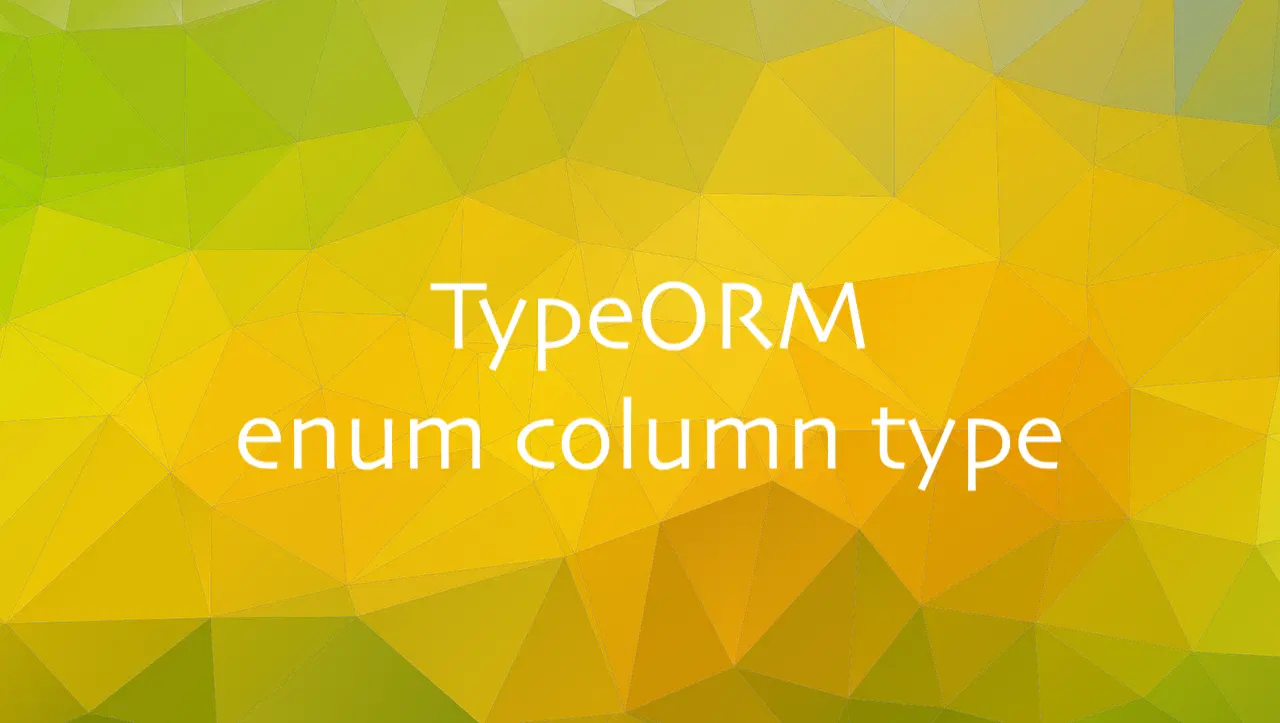
If you’re working with PostgreSQL or MySQL and making use of TypeORM in your code then you can add a column with the ENUM (enumerated) data type like so:
// book.entity.ts
import {
Entity,
PrimaryGeneratedColumn,
Column,
} from 'typeorm'
export enum BookFormat {
HARDCOVER = 'hardcover',
PAPERBACK = 'paperback',
DIGITAL = 'digital'
}
@Entity()
export class Book {
@PrimaryGeneratedColumn()
id: number;
@Column()
title: string;
@Column({
type: 'enum',
enum: BookFormat,
default: BookFormat.DIGITAL
})
format: BookFormat
}
This entity will create a table named books with a format column look as shown below (If you’re using Postgres, you will get the same result as mine. If you’re using MySQL, the outcome is almost the same):
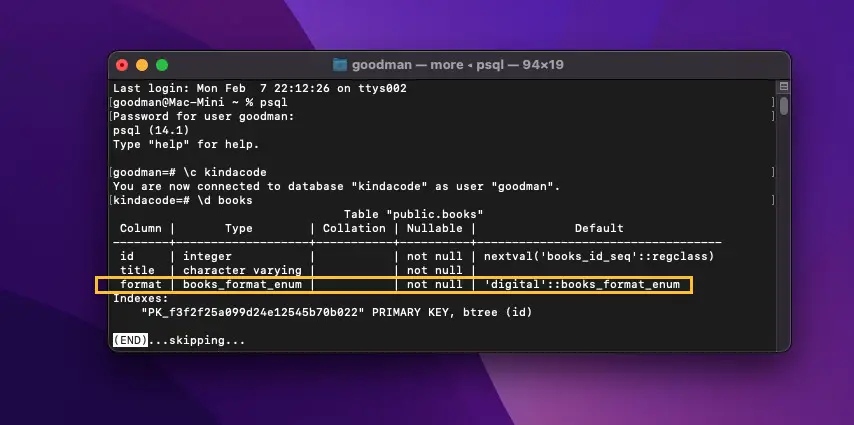
In case you want to use an array with enum values:
import {
Entity,
PrimaryGeneratedColumn,
Column,
} from 'typeorm'
export type BookFormat = "hardcover" | "paperback" | "digital";
@Entity({name: 'books'})
export class Book {
@PrimaryGeneratedColumn()
id: number;
@Column()
title: string;
@Column({
type: 'enum',
enum: ["hardcover", "paperback", "digital"],
default: "digital"
})
format: BookFormat
}
Further reading:
- TypeORM: Property ‘id’ has no initializer and is not definitely assigned in the constructor
- TypeORM: Adding created_at and updated_at columns
- Node.js: How to Compress a File using Gzip format
- Best open-source ORM and ODM libraries for Node.js
- 2 Ways to Rename a Table in PostgreSQL
You can also check out our database topic page for the latest tutorials and examples.