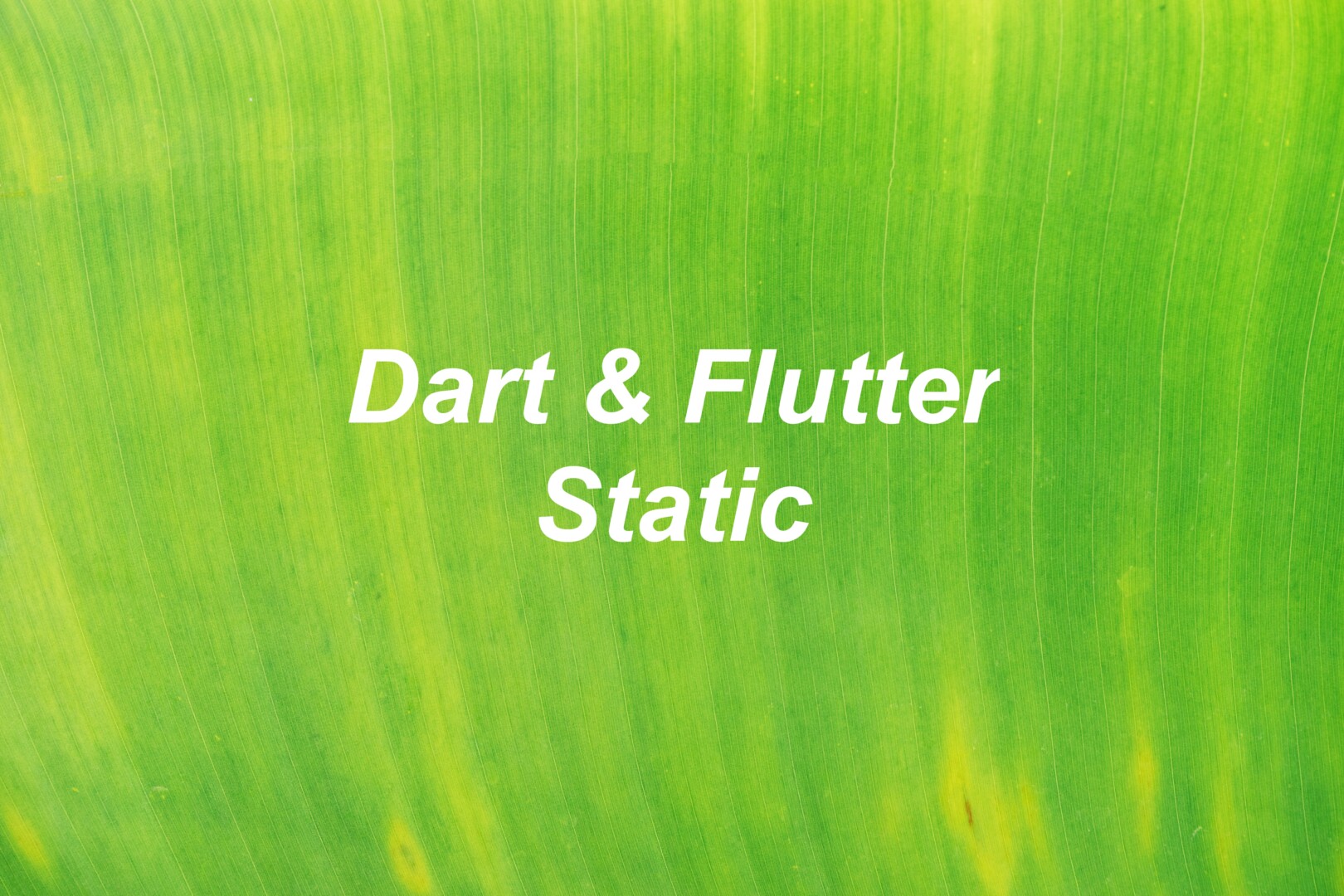
In Dart and Flutter, a static method (or a static function) is a method defined as a member of a class but can be directly called without creating an object instance via the constructor. In other words, a static method is a port of a class instead of being a part of a specific instance.
Syntax:
class MyClass {
static void method1(){
/* ... */
}
static String method2(){
/*...*/
return "Some string";
}
}
// Call static methods
void main(){
MyClass.method1();
String s = MyClass.method2();
}
A Working Example:
// main.dart
class ExampleClass {
static void sayHello() {
print("Hello buddy, welcome to KindaCode.com");
}
static int sum(int input1, int input2) {
return input1 + input2;
}
}
void main() {
ExampleClass.sayHello();
final sum = ExampleClass.sum(10, 11);
print(sum);
}
Output:
Hello buddy, welcome to KindaCode.com
21
You can see how static methods are used in this practical guide: Flutter & SQLite: CRUD Example.
Further reading:
- Dart: Converting a List to a Map and Vice Versa
- Using Cascade Notation in Dart and Flutter
- Dart: Convert Timestamp to DateTime and vice versa
- Sorting Lists in Dart and Flutter (5 Examples)
- Flutter: Caching Network Images for Big Performance gains
- Dart: Sorting Entries of a Map by Its Values
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.