This article walks you through a couple of examples of viewing PDF (.pdf) documents from within a Flutter app. We are going to use the following PDF file for testing purposes:
https://www.kindacode.com/wp-content/uploads/2021/07/test.pdf
Using advance_pdf_viewer plugin
This package helps you view both local and network pdf files with ease. You can install it by executing the following command:
flutter pub add advance_pdf_viewer
Example Preview
Here’s what we’re going to make:
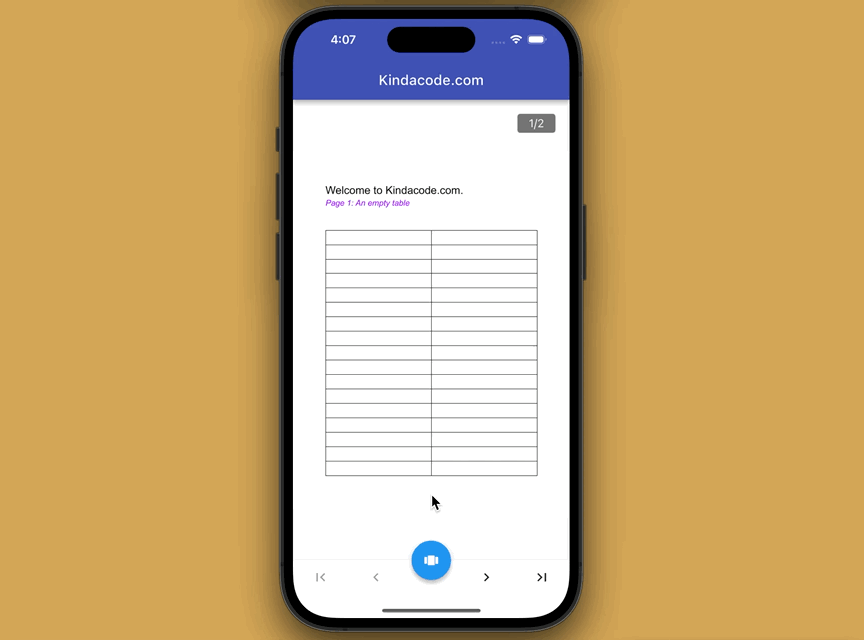
The beautiful control bar in the demo video above was added automatically by the plugin.
The Complete Code
// main.dart
import 'package:flutter/material.dart';
import 'package:advance_pdf_viewer/advance_pdf_viewer.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const HomePage());
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
bool _isLoading = true;
late PDFDocument _pdf;
void _loadFile() async {
// Load the pdf file from the internet
_pdf = await PDFDocument.fromURL(
'https://www.kindacode.com/wp-content/uploads/2021/07/test.pdf');
setState(() {
_isLoading = false;
});
}
@override
void initState() {
super.initState();
_loadFile();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: _isLoading
? const Center(child: CircularProgressIndicator())
: PDFViewer(document: _pdf)),
);
}
}
If you want to render an offline PDF document from your assets folder, use this:
_pdf = await PDFDocument.fromAsset('assets/test.pdf'); // Replace test.pdf with your own file name
In this case, don’t forget to add an asset section in your pubspec.yaml file:
flutter:
uses-material-design: true
assets:
- assets/
Using url_launcher plugin
This plugin will open the PDF file with the default web browser of the device.
Installation
flutter pub add url_launcher
Add required permissions
For iOS, add the following to your ios/Runner/Info.plist file:
<key>LSApplicationQueriesSchemes</key>
<array>
<string>https</string>
</array>
For Android, modify your AndroidManifest.xml file:
<queries>
<intent>
<action android:name="android.intent.action.VIEW" />
<data android:scheme="https" />
</intent>
</queries>
Screenshot:
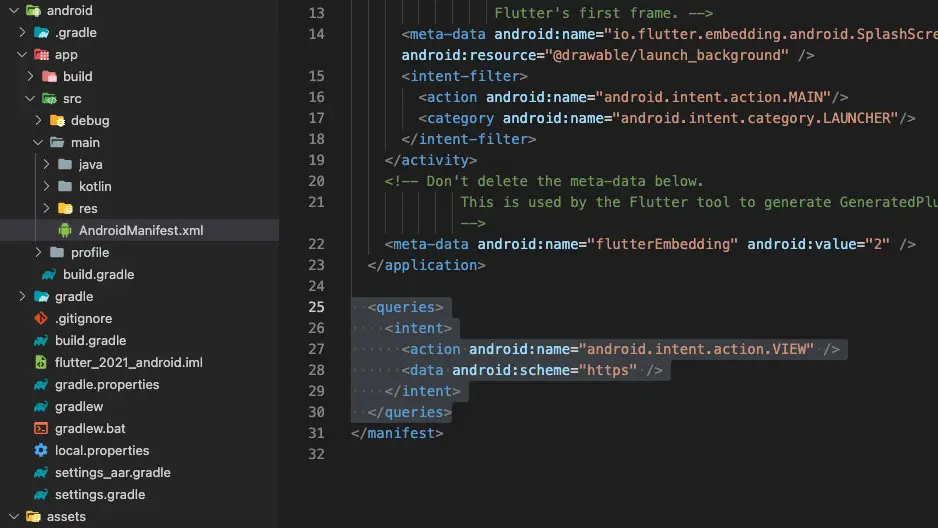
Example Preview
A demo is worth more than a thousand words:
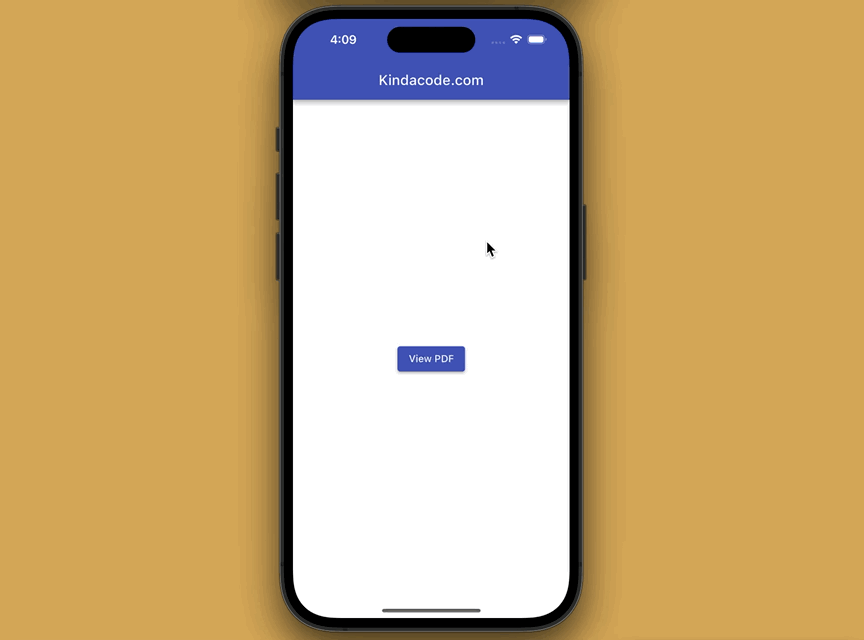
The Complete Code
// main.dart
import 'package:flutter/material.dart';
import 'package:url_launcher/url_launcher_string.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const HomePage());
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
void _viewFile() async {
const url = 'https://www.kindacode.com/wp-content/uploads/2021/07/test.pdf';
try {
await launchUrlString(url);
} catch (err) {
debugPrint('Something went wrong');
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: ElevatedButton(
onPressed: _viewFile,
child: const Text('View PDF'),
),
),
);
}
}
Conclusion
We’ve gone over a few examples of reading PDF files from within an app. If you are working with other file types, take a look at the following tutorials:
- Flutter: Load and display content from CSV files
- Flutter: How to Read and Write Text Files
- How to encode/decode JSON in Flutter
- How to read data from local JSON files in Flutter
If you’d like to explore more new and interesting stuff in Flutter:
- Using GetX (Get) for Navigation and Routing in Flutter
- Using GetX (Get) for State Management in Flutter
- Flutter StreamBuilder examples (null safety)
- Flutter FutureBuilder example (null safety)
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.