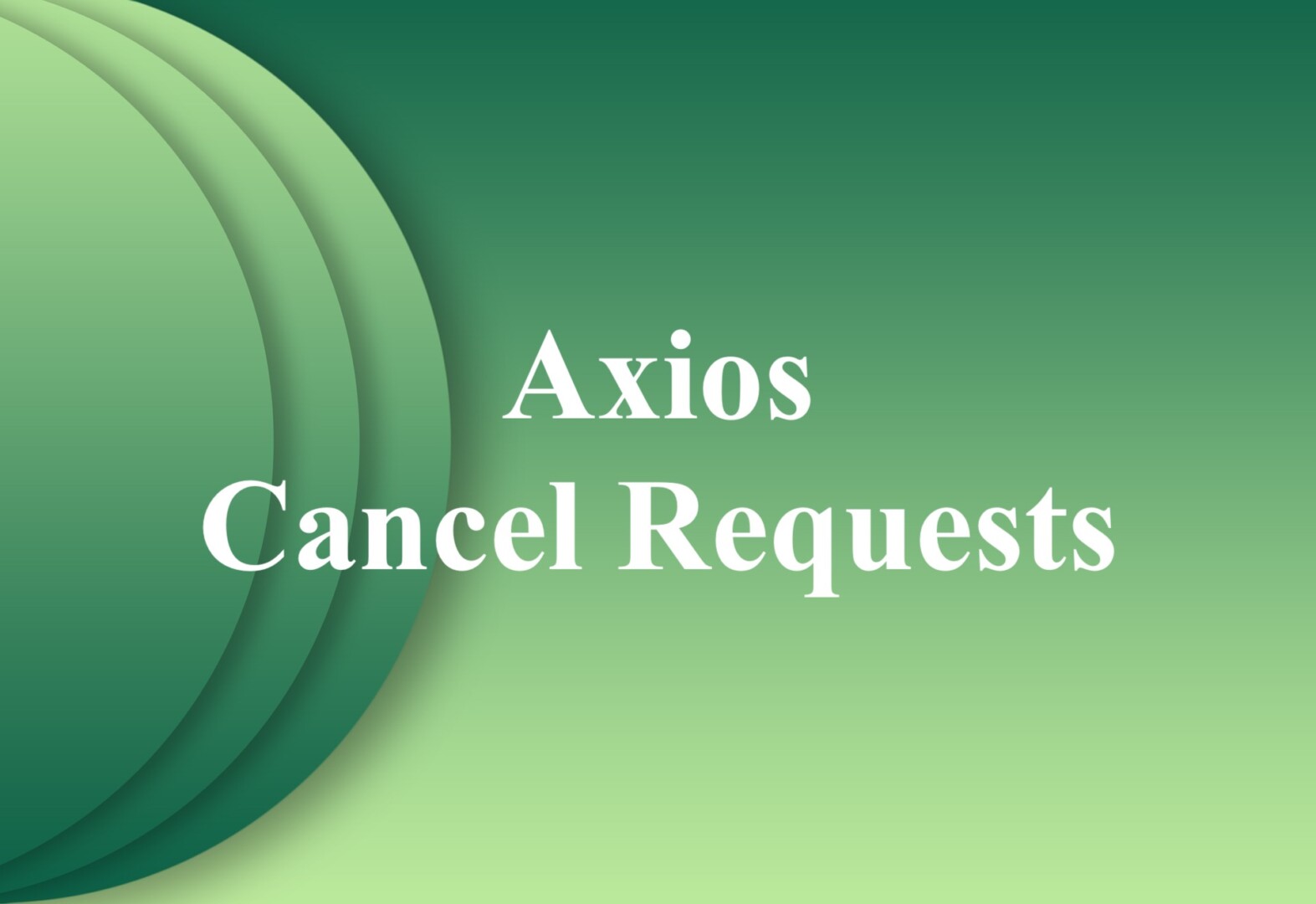
This concise and straight-to-the-point article shows you 2 distinct ways to cancel a recently sent request in Axios. Without any further ado, let’s get started.
Using AbortController
If you’re developing a project with a new version of Axios, this approach is encouraged. Below are the steps:
1. Create a controller:
const controller = new AbortController();
2. When making a request, set the signal option to controller.signal:
try {
const response = await axios.get('https://fake-api.kindacode.com', {
signal: controller.signal,
});
console.log(response);
} catch (error: any) {
if (axios.isCancel(error)) {
console.log('Request canceled', error.message);
} else {
console.log(error);
}
}
3. Calle controller.abort() to cancel the request (this can be done programmatically or by your user’s action):
controller.abort();
This will trigger the catch block and print “Request canceled”.
Using CancelToken
If you’re using an old version of Axios (< 0.22), this approach is a good choice. Otherwise, go with the first solution.
The steps:
1. Create the source.
const CancelToken = axios.CancelToken;
const source = CancelToken.source();
2. Set the cancelToken property to source.token when making a request:
try {
const response = await axios.get('https://fake-api.kindacode.com', {
cancelToken: source.token,
});
console.log(response);
} catch (error: any) {
if (axios.isCancel(error)) {
console.log('Request canceled', error.message);
} else {
console.log(error);
}
}
3. You can cancel the request by triggering the source.calcel() method:
source.cancel('Operation canceled by the user.');
This will throw a Cancel error and you can catch it within the catch() block.
That’s it. Continue learning more about Axios by taking a look at the following articles:
- Axios: How to Set Timeouts for Requests
- React: How to Upload Multiple Files with Axios
- Axios: Set Headers in GET/POST Requests
- Axios: Passing Query Parameters in GET/POST Requests
- 7 Best Open-Source HTTP Request Libraries for Node.js
- 5 Best Open-Source HTTP Request Libraries for React
You can also check out our Javascript category page, TypeScript category page, Node.js category page, and React category page for the latest tutorials and examples.