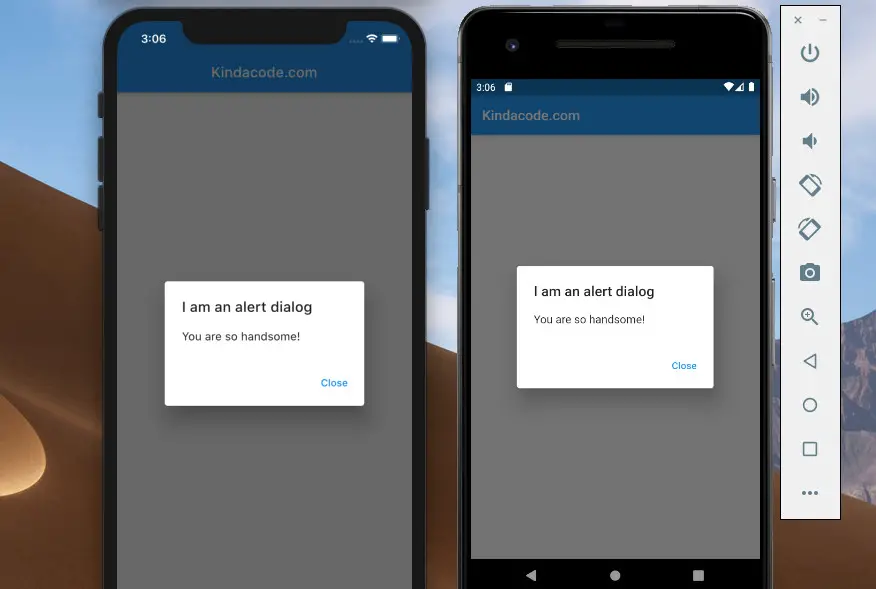
AlertDialog is a built-in widget in Flutter that has an optional title, optional content, and an optional list of actions.
In general, the AlertDialog widget is used with the showDialog() function (a built-in function of Flutter) like so:
showDialog(
context: context,
builder: (_) => AlertDialog(/*...*/)
);
To close that dialog, just use:
Navigator.of(context).pop()
Example
The tiny app we are going to build contains a button in the center of the screen. When the button gets pressed, a dialog with a Close button will show up. You can make the dialog go away by hitting the Close button or tapping outside the dialog.
Preview:
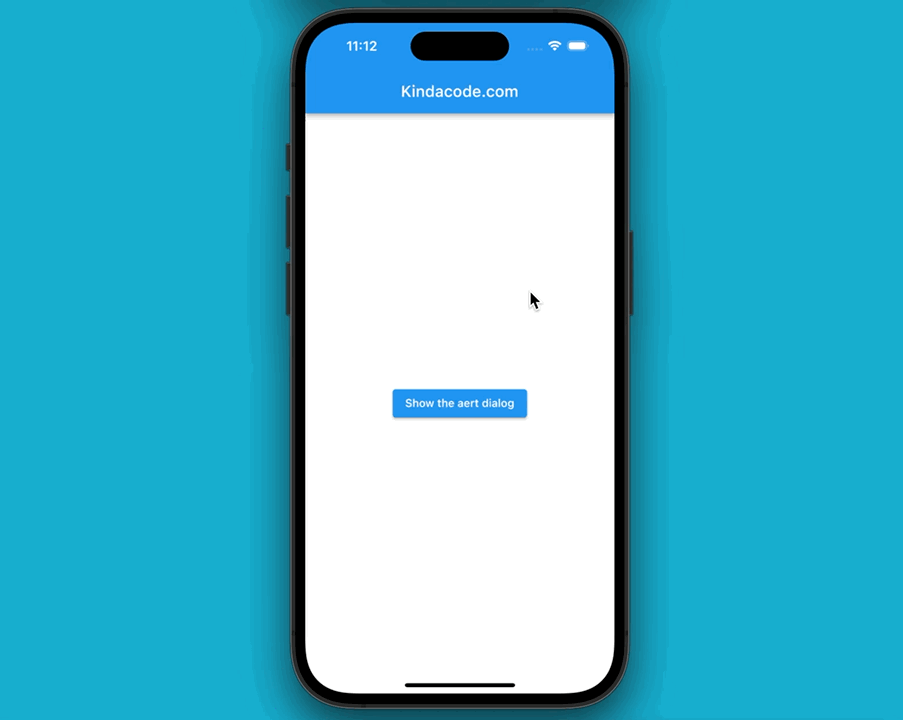
This is the function that is called when the button is pressed:
void _show(BuildContext ctx) {
showDialog(
context: ctx,
builder: (_) => AlertDialog(
title: const Text('I am an alert dialog'),
content: const Text('You are so handsome!'),
actions: [
TextButton(
onPressed: () => Navigator.of(ctx).pop(),
child: const Text('Close '))
],
));
}
The full code in main.dart:
// kindacode.com
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// Show the alert dialog
// The context is the context of the widget that calls this method
void _show(BuildContext ctx) {
showDialog(
context: ctx,
builder: (_) => AlertDialog(
title: const Text('I am an alert dialog'),
content: const Text('You are so handsome!'),
actions: [
TextButton(
onPressed: () => Navigator.of(ctx).pop(),
child: const Text('Close '))
],
));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
// This button will show the alert dialog
child: ElevatedButton(
child: const Text('Show the aert dialog'),
onPressed: () => _show(context),
),
),
);
}
}
That’s it. Further reading:
- How to Make a Confirm Dialog in Flutter
- Flutter Cupertino Button – Tutorial and Examples
- Flutter: SliverGrid example
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
- Write a simple BMI Calculator with Flutter (Null Safety)
- Flutter StreamBuilder examples (null safety)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.