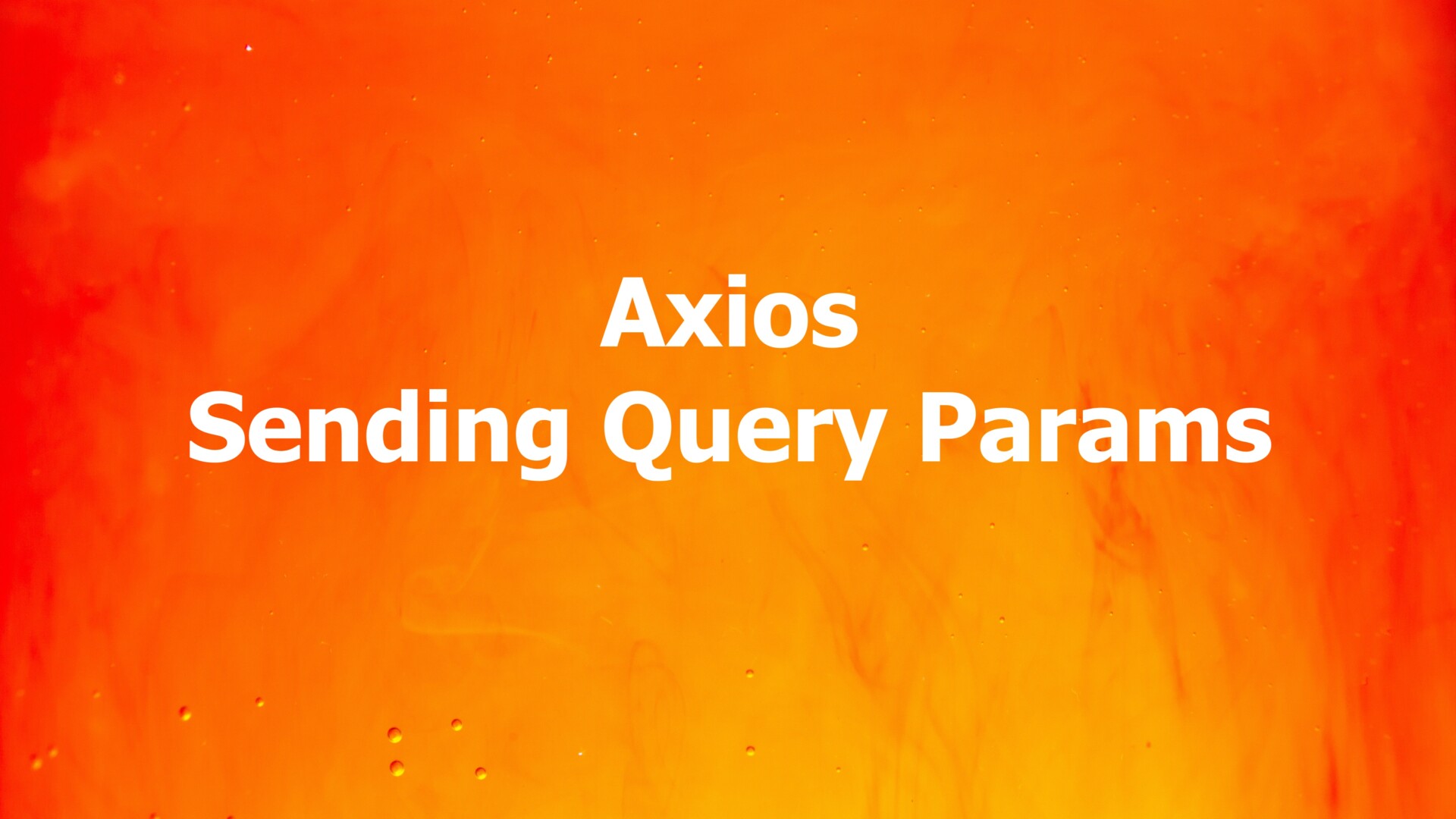
This quick and at-a-glance article shows you how to pass query parameters in a GET or POST request when using Axios, a very popular Javascript HTTP library.
Passing Query Params in a GET Request
Axios syntax for GET is:
axios.get(url: string, config?: AxiosRequestConfig<any> | undefined)
Therefore, you can add your query params object in the second argument, like so:
const res = await axios.get('https://fake-api.kindacode.com/tutorials', {
params: {
paramOne: 'one',
paramTwo: 'two',
paramThree: 'three',
foo: 1,
bar: 2,
},
});
It will make a GET request with 5 query params:
https://fake-api.kindacode.com/tutorials?paramOne=one¶mTwo=two¶mThree=three&foo=1&bar=2
Passing Query Params in a POST Request
Axios syntax for POST is:
axios.post(
url: string,
data?: {} | undefined,
config?: AxiosRequestConfig<{}> | undefined
)
So that you can send query params in a POST request through the third argument object, like this:
const res = await axios.post(
'https://fake-api.kindacode.com/tutorials',
{
// data to sent to the server - post body
// it can be an empty object
},
{
// specify query parameters
params: {
paramOne: 'one',
paramTwo: 'two',
paramThree: 'three',
},
}
);
The code above will post an empty body with 3 query params:
https://fake-api.kindacode.com/tutorials?paramOne=one¶mTwo=two¶mThree=three
Further reading:
- 5 Best Open-Source HTTP Request Libraries for React
- 7 Best Open-Source HTTP Request Libraries for Node.js
- How to fetch data from APIs with Axios and Hooks in React
- Javascript: Convert Relative URLs to Absolute URLs
- Node.js: How to Ping a Remote Server/ Website
- Nodemon: Automatically Restart a Node.js App on Crash
You can also check out our Javascript category page, TypeScript category page, Node.js category page, and React category page for the latest tutorials and examples.