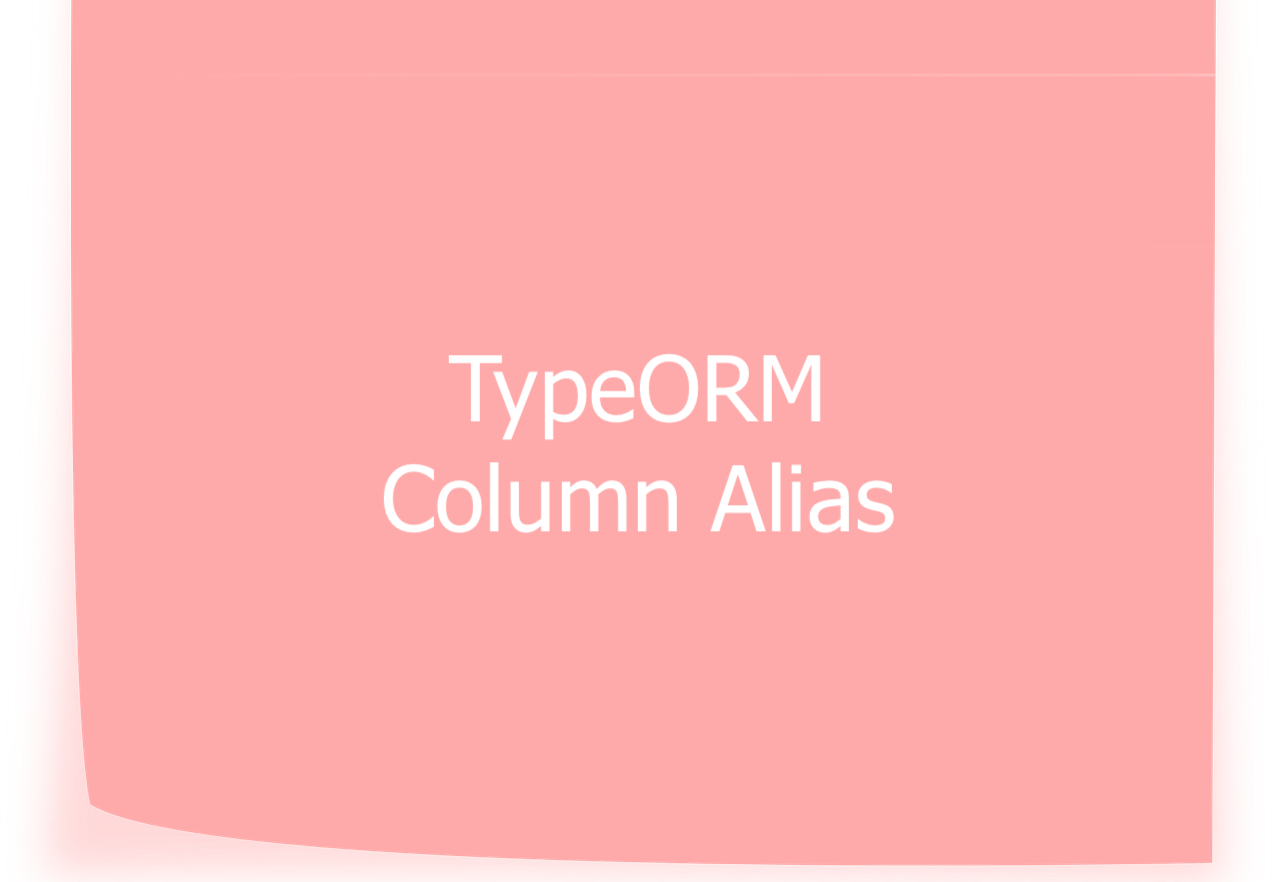
This succinct tutorial shows you how to use column aliases to assign temporary names to columns when selecting data with TypeORM. The point here is the AS keyword. Let’s study a couple of examples for more clarity.
User entity:
// KindaCode.com
// User entity
import { Entity, PrimaryGeneratedColumn, Column} from 'typeorm';
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column({nullable: true})
age: number;
}
Using column aliases within a query builder to retrieve all users:
const userRepository = myDataSource.getRepository(User);
const users = await userRepository
.createQueryBuilder('user')
.select([
'user.id AS user_id',
'user.name AS user_name',
'user.age as user_age',
])
.getRawMany();
console.log(users);
Your output will look similar to this:
[
{ user_id: 1, user_name: 'User 1', user_age: 20 },
{ user_id: 2, user_name: 'User 2', user_age: 30 },
{ user_id: 3, user_name: 'User 3', user_age: 40 }
]
TypeORM is evolving quickly and there is much to be learned. Make your skills keep up with the times by taking a look at the following articles:
- TypeORM: Selecting DISTINCT Values
- Aggregation Operations in TypeORM (Sum, Avg, Min, Max, Count)
- TypeORM: Using LIKE Operator (2 Examples)
- TypeORM Upsert: Update If Exists, Create If Not Exists
- TypeORM: Selecting Rows with Null Values
You can also check out our database topic page for the latest tutorials and examples.